Introduction of Programming methodology
Programming methodology refers to the systematic approach used in designing, developing, testing, and maintaining software applications. It encompasses the principles, techniques, and tools that software developers use to create high-quality, efficient, and maintainable software. A well-defined programming methodology helps ensure that software projects are completed on time, within budget, and with the desired functionality and quality.
Here’s an introduction to programming methodology, including key concepts and different approaches:
Key Concepts in Programming Methodology
- Problem Definition:
- Clearly define the problem that the software is intended to solve. This involves understanding the requirements, constraints, and goals of the project.
- Requirements gathering is a crucial step, often involving stakeholders to ensure that the software meets the needs of its users.
- Algorithm Design:
- Design algorithms that provide step-by-step instructions for solving the problem.
- Algorithms should be efficient, correct, and easy to understand and implement.
- Data Structures:
- Choose appropriate data structures to organize and manage data efficiently.
- Common data structures include arrays, linked lists, stacks, queues, trees, and graphs.
- Code Implementation:
- Translate algorithms and data structures into code using a programming language.
- Writing clean, readable, and maintainable code is essential for long-term project success.
- Testing and Debugging:
- Test the software to ensure it works as expected and meets the requirements.
- Debugging involves identifying and fixing errors or bugs in the code.
- Documentation:
- Provide documentation for the code, including comments, user manuals, and technical specifications.
- Good documentation makes it easier for other developers to understand and maintain the code.
- Maintenance:
- Maintain the software by fixing bugs, adding new features, and updating it to remain compatible with changing technologies and user needs.
- Software maintenance is often the most time-consuming phase of a software project.
Steps Involved in programming
Programming involves a series of steps to develop software applications systematically and efficiently. Each step in the process builds upon the previous one, ensuring that the final product meets the desired requirements and functions correctly. Here’s a detailed look at the steps involved in programming:
1. Problem Definition
Description:
This initial step involves clearly defining the problem that the software aims to solve. It requires a thorough understanding of the project’s requirements, constraints, and goals.
Activities:
- Identify the problem and understand the needs of the end users.
- Gather requirements by consulting with stakeholders and users.
- Define the scope of the project, including functional and non-functional requirements.
- Document requirements clearly to guide subsequent steps.
Outcome:
A well-defined problem statement and a requirements document that outlines the project’s objectives and scope.
2. Planning
Description:
Planning involves creating a roadmap for the project, determining the resources, timeline, and tasks required to complete the project successfully.
Activities:
- Break down the project into smaller, manageable tasks.
- Estimate the time and resources needed for each task.
- Create a project timeline with milestones and deadlines.
- Identify potential risks and plan for their mitigation.
Outcome:
A project plan that includes a timeline, resource allocation, and risk management strategies.
3. Algorithm Design
Description:
Algorithm design involves creating a logical sequence of steps to solve the problem identified in the first step. It serves as a blueprint for the code.
Activities:
- Develop algorithms that outline the step-by-step procedures to achieve the desired functionality.
- Consider efficiency, correctness, and simplicity in algorithm design.
- Use flowcharts or pseudocode to represent the algorithms visually.
Outcome:
A set of well-defined algorithms that provide a clear roadmap for code implementation.
4. Data Structure Selection
Description:
Choosing the appropriate data structures is crucial for efficient data management and manipulation within the program.
Activities:
- Analyze the data requirements of the application.
- Select appropriate data structures, such as arrays, linked lists, stacks, queues, trees, and graphs.
- Consider the trade-offs in terms of complexity, performance, and memory usage.
Outcome:
A decision on the data structures to be used, which will support efficient data storage and retrieval.
5. Code Implementation
Description:
This step involves translating the algorithms and data structures into executable code using a programming language.
Activities:
- Write the code, ensuring it is clean, readable, and well-documented.
- Follow coding standards and best practices to maintain consistency and quality.
- Use version control systems like Git to manage code changes and collaborate with other developers.
Outcome:
A functional codebase that implements the specified algorithms and data structures.
6. Testing and Debugging
Description:
Testing and debugging are crucial steps to ensure the software works as intended and is free of errors.
Activities:
- Perform unit testing to verify the functionality of individual components.
- Conduct integration testing to ensure components work together seamlessly.
- Use debugging tools to identify and fix errors in the code.
- Conduct user acceptance testing (UAT) to validate that the software meets user expectations.
Outcome:
A tested and debugged application that meets the specified requirements and performs reliably.
7. Documentation
Description:
Documentation provides essential information about the software, including how it was developed, how it works, and how to use it.
Activities:
- Write internal documentation within the code, such as comments and annotations.
- Develop user manuals and technical guides for end-users and developers.
- Maintain documentation to reflect changes and updates in the software.
Outcome:
Comprehensive documentation that supports software maintenance, usage, and further development.
8. Deployment
Description:
Deployment involves releasing the software to users and making it available for use in the intended environment.
Activities:
- Prepare the deployment environment, including hardware, software, and network configurations.
- Install and configure the software for end-users.
- Provide training and support to users as needed.
Outcome:
A successfully deployed application that is ready for use by end-users.
9. Maintenance and Updates
Description:
Software maintenance involves making improvements, fixing bugs, and updating the software to adapt to changing requirements or technologies.
Activities:
- Monitor the software for issues and performance metrics.
- Implement bug fixes, patches, and updates as needed.
- Add new features and enhancements based on user feedback and evolving requirements.
Outcome:
An updated and well-maintained application that continues to meet user needs and adapts to changes in technology or business requirements.
Each step in the programming process plays a vital role in ensuring that the software is well-designed, functional, and reliable. By following these steps systematically, developers can create high-quality software that meets the needs of users and stakeholders.
Algorithm
An algorithm is a well-defined, step-by-step procedure or set of rules designed to perform a specific task or solve a particular problem. It serves as a blueprint for writing a program or creating a system to achieve the desired outcome. Algorithms are fundamental to computer science and are used in a wide range of applications, from simple tasks like sorting numbers to complex operations like data encryption and artificial intelligence.
Characteristics of Algorithm
An algorithm is a step-by-step procedure designed to solve a problem or perform a specific task. For an algorithm to be effective and useful, it must exhibit certain characteristics. Here are the key characteristics of an algorithm:
1. Finiteness
Definition:
An algorithm must have a finite number of steps and should terminate after a specific number of operations. It should not go into an infinite loop.
Explanation:
An algorithm is considered finite if it concludes after executing a defined sequence of instructions, regardless of input size. Each step must be well-defined and eventually lead to the algorithm’s termination. This ensures that the algorithm produces a result in a reasonable amount of time.
Example:
An algorithm to find the sum of the first n
natural numbers should have a finite number of steps and provide the sum as the output. It should not continue indefinitely.
2. Definiteness
Definition:
Each step of an algorithm must be precisely and unambiguously defined. The instructions should be clear and specific, leaving no room for interpretation.
Explanation:
Definiteness ensures that every instruction in the algorithm is exact, leaving no ambiguity about what actions to perform. This means that each step is explicitly detailed, so it is understandable and executable without confusion.
Example:
An algorithm that sorts a list should clearly specify the method of sorting (e.g., bubble sort, quicksort) and define the actions taken in each step to rearrange elements in the desired order.
3. Input
Definition:
An algorithm must have zero or more inputs that are supplied externally. These inputs are the initial data needed to execute the algorithm.
Explanation:
The inputs provide the necessary data for the algorithm to process. An algorithm can operate on zero inputs (e.g., generating a constant value) or multiple inputs, which it processes to produce the output.
Example:
A search algorithm might take a list of numbers and a target value as inputs. Without these inputs, the algorithm cannot perform its function.
4. Output
Definition:
An algorithm must produce at least one output. The output is the result or solution obtained after executing the algorithm’s steps.
Explanation:
The output is the result produced by the algorithm after processing the inputs. An algorithm should generate meaningful and correct results that satisfy the problem requirements.
Example:
An algorithm that calculates the factorial of a number should output the factorial value as the result.
5. Effectiveness
Definition:
An algorithm’s steps must be basic enough to be carried out precisely in a finite amount of time using available resources.
Explanation:
Effectiveness ensures that each step of the algorithm is simple and feasible. Each operation should be executable with basic arithmetic or logical operations, making the algorithm practical and implementable.
Example:
An algorithm that calculates the greatest common divisor (GCD) of two numbers should use straightforward arithmetic operations, ensuring that the steps are simple and directly executable.
Program
A program is a set of instructions written in a specific programming language that a computer can execute to perform a particular task or solve a specific problem. It is a concrete implementation of an algorithm, which transforms inputs into outputs through a series of computational steps. Programs range from simple scripts that perform basic tasks to complex systems like operating systems and software applications.
Key Characteristics of a Program
- Instructions:
- A program consists of a sequence of instructions that specify the operations to be performed.
- Instructions can include arithmetic operations, data manipulation, conditional logic, loops, and function calls.
- Programming Language:
- Programs are written in programming languages, which provide the syntax and semantics for writing code.
- Programming languages can be high-level (e.g., Python, Java, C++) or low-level (e.g., Assembly, Machine Code).
- Execution:
- Programs are executed by computers, which follow the instructions step-by-step to perform tasks.
- The execution process involves translating the program’s code into machine language through a compiler or interpreter.
- Inputs and Outputs:
- Programs often take inputs, process them according to the instructions, and produce outputs.
- Inputs can be provided by users, other programs, or external data sources. Outputs can be displayed to users or stored for further use.
Difference Between Program and Algorithm
The concepts of a program and an algorithm are fundamental to computer science and programming, but they refer to different aspects of solving problems with computers. Here’s a detailed comparison highlighting the key differences between a program and an algorithm:
- Algorithm:
- An algorithm is a logical sequence of steps that describes how to solve a specific problem. It is conceptual and serves as a blueprint for problem-solving, independent of any programming language.
- Example: An algorithm for finding the maximum number in a list of integers.
- Program:
- A program is a practical implementation of an algorithm, written in a specific programming language, that can be executed by a computer to perform a task.
- Example: A Python program that implements the algorithm to find the maximum number in a list.
Feature | Algorithm | Program |
---|---|---|
Definition | An algorithm is a step-by-step, logical procedure for solving a problem or performing a task. | A program is a set of coded instructions written in a programming language to execute an algorithm. |
Nature | Abstract and conceptual. | Concrete and implementable. |
Representation | Expressed in pseudocode or flowcharts. | Written in a specific programming language (e.g., Python, Java, C++). |
Purpose | To describe the logic and sequence of steps to solve a problem. | To implement an algorithm on a computer to perform a task. |
Input/Output | Algorithms focus on the logic of transforming inputs to outputs. | Programs handle actual data input and output operations. |
Execution | Cannot be executed directly by a computer. | Executed directly by a computer. |
Components | Includes steps like iteration, selection, and logical flow. | Includes components like data structures, control structures, and libraries. |
Abstraction Level | High-level, independent of any programming language. | Low-level, specific to a programming language and hardware. |
Modification | Easier to modify conceptually without worrying about syntax. | Modifications require changes in syntax and possibly re-testing of code. |
Complexity | Complexity is measured in terms of time and space efficiency. | Complexity involves code readability, maintainability, and runtime performance. |
Difference Between Flowchart and Algorithm
Flowcharts and algorithms are both tools used to describe and represent the logic and steps involved in solving a problem or performing a task, but they do so in different ways. Here’s a detailed comparison between flowcharts and algorithms:
- Flowchart:
- Definition: A flowchart is a diagrammatic representation of an algorithm or process, using various symbols to denote different types of operations and the flow of control.
- Representation: Uses graphical symbols like ovals for start/end, rectangles for processes, diamonds for decisions, and arrows for the flow of control.
- Algorithm:
- Definition: An algorithm is a written or symbolic description of a step-by-step procedure for solving a problem or performing a task.
- Representation: Uses text or pseudocode to describe the sequence of operations and decisions needed.
Feature | Flowchart | Algorithm |
---|---|---|
Definition | A flowchart is a visual representation of the sequence of steps and decisions needed to solve a problem. | An algorithm is a step-by-step, logical procedure for solving a problem or performing a task. |
Representation | Uses graphical symbols and arrows to represent steps, decisions, and the flow of control. | Uses textual or pseudocode descriptions to outline the steps. |
Visualization | Provides a visual layout of the process, making it easy to see the flow and interactions. | Provides a textual or symbolic representation that focuses on the logic and sequence of steps. |
Clarity | Offers a clear, visual depiction of the process, which can be easier to understand at a glance. | Offers a detailed, logical description that may require more effort to visualize and understand. |
Usage | Often used to illustrate and design processes, workflows, and systems. | Often used to design and specify the steps involved in solving a problem or implementing a solution. |
Components | Includes symbols like ovals (start/end), rectangles (process steps), diamonds (decisions), and arrows (flow). | Includes keywords and constructs such as steps, loops, conditionals, and function calls. |
Complexity | Can become complex and difficult to read with intricate processes or large numbers of steps. | Can become verbose and difficult to follow with very detailed or lengthy procedures. |
Modification | Changes are made by updating the visual representation, which may require redrawing parts of the flowchart. | Changes are made by editing the textual or pseudocode description, which may be simpler to modify. |
Focus | Focuses on the flow of control and interactions between steps. | Focuses on the logical sequence and detailed steps for solving the problem. |
Tools | Typically created using drawing tools or flowchart software. | Typically written using text editors or pseudocode tools. |
Symbols Used in Flow Charts
1. Terminator symbol:
Meaning: the start or end of a process
An oval symbol marks the first and last steps of a process. You can include “start” or “end” in the oval to highlight their order. You can use more than one oval for processes with multiple outcomes. Labeling these ovals with numbers or letters helps you track each endpoint in a complex user journey.
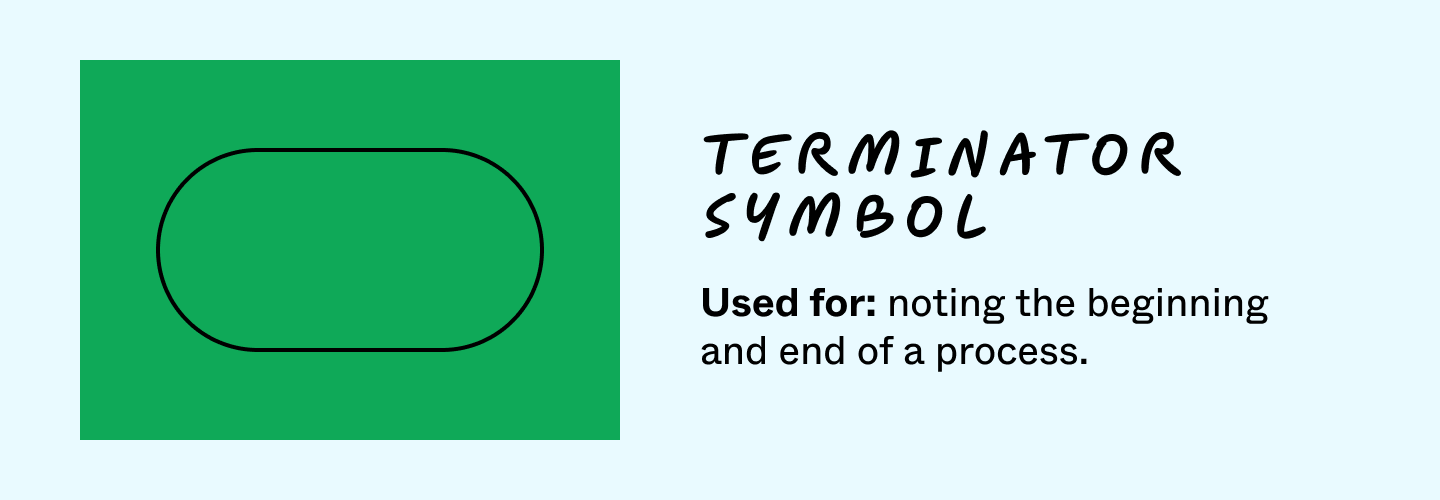
2. Action symbol
Meaning: the actions or steps needed to carry out a process
A rectangular action symbol represents any step in a process. As a result, some teams call it a process symbol. These steps connect basic tasks or actions needed to reach an outcome. Manual actions and automatic steps both use this symbol, making it one of the most popular symbols in flowcharting.
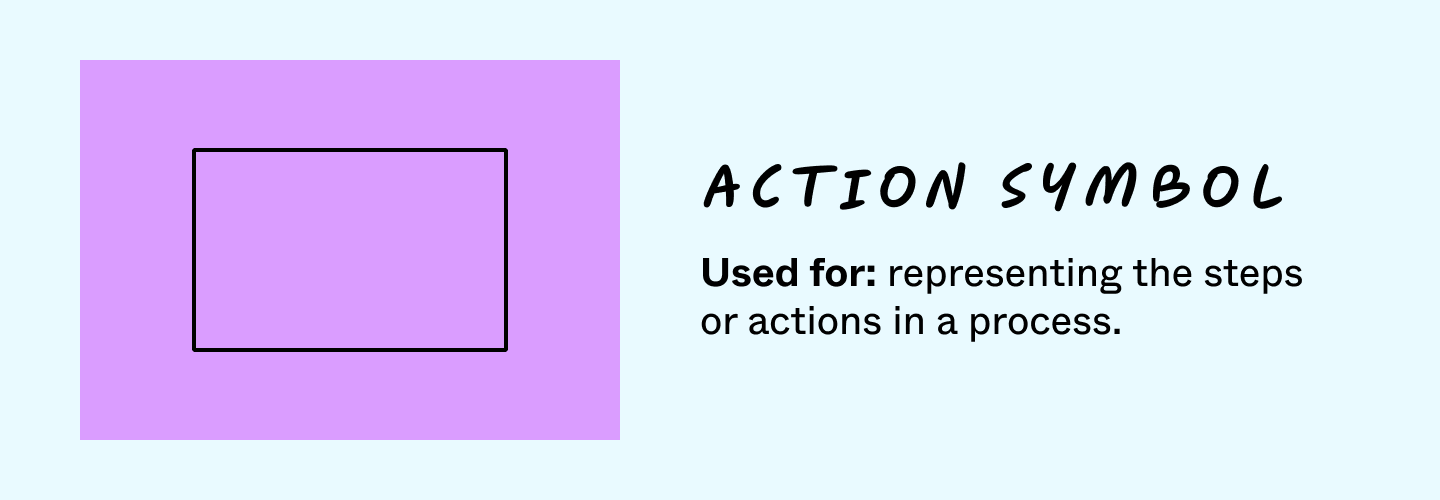
3. Decision symbol
Diamond markers symbolize decisions users make at crossroads in a flowchart. You can place decision markers at simple forks in the road or points leading to multiple paths. More often than not, decision markers represent a true/false or yes/no question.
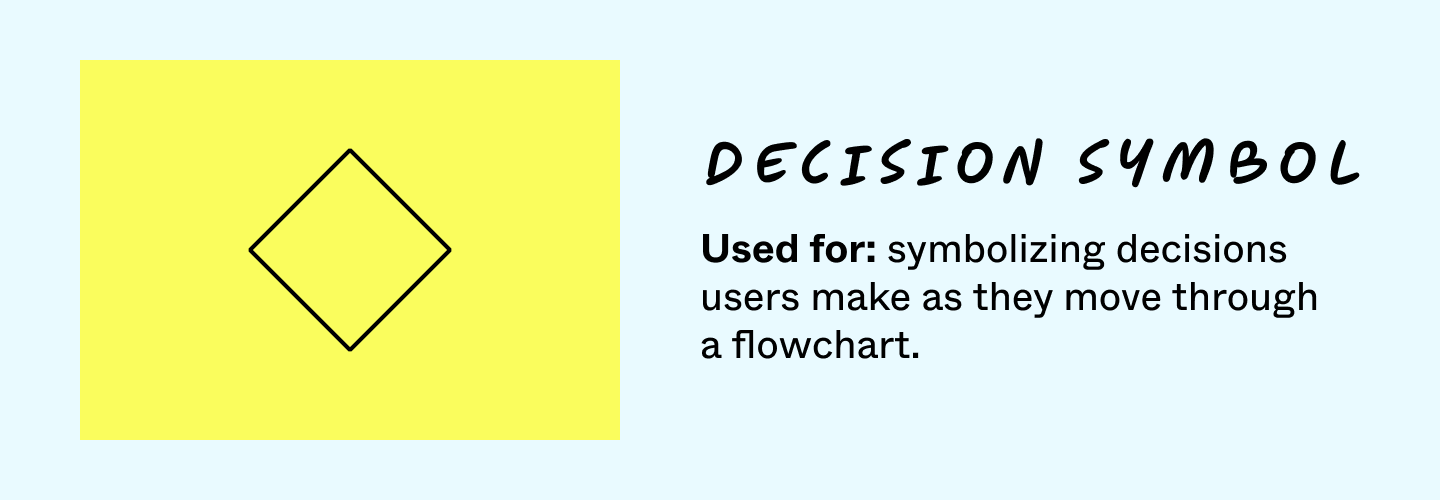
4. Input and output symbol
Meaning: data or finished products entering and leaving a process
Input and output symbols reveal when goods or data enter and leave a system. Sometimes called the data symbol, this icon shows how resources are used or generated. Inputs refer to something a user enters, while outputs can mean any data or products built by a system.
For example, customer orders and payments represent a system input. Once you process this input, your system gives an output, such as an order delivery. When adding this symbol, specify whether it means an input or an output.
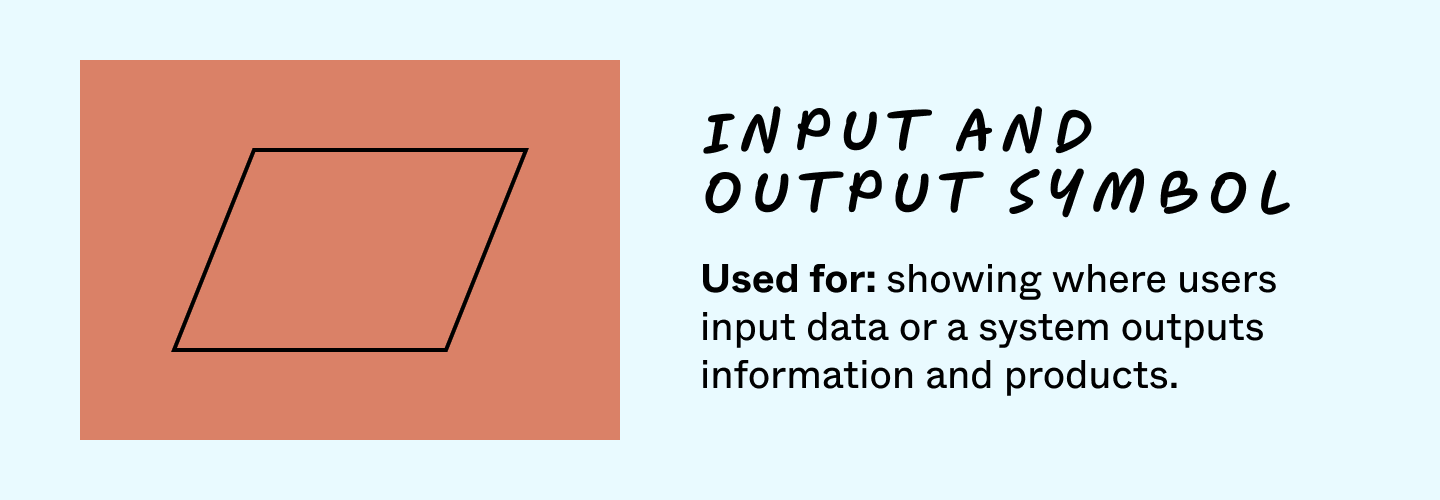
5. On-page connector symbol
Meaning: points where flows end and resume on different parts of a chart
On-page connectors link different elements on a page, and can replace long arrows on a complex flowchart. Keep track of each symbol by placing the same letter or number inside two connectors.
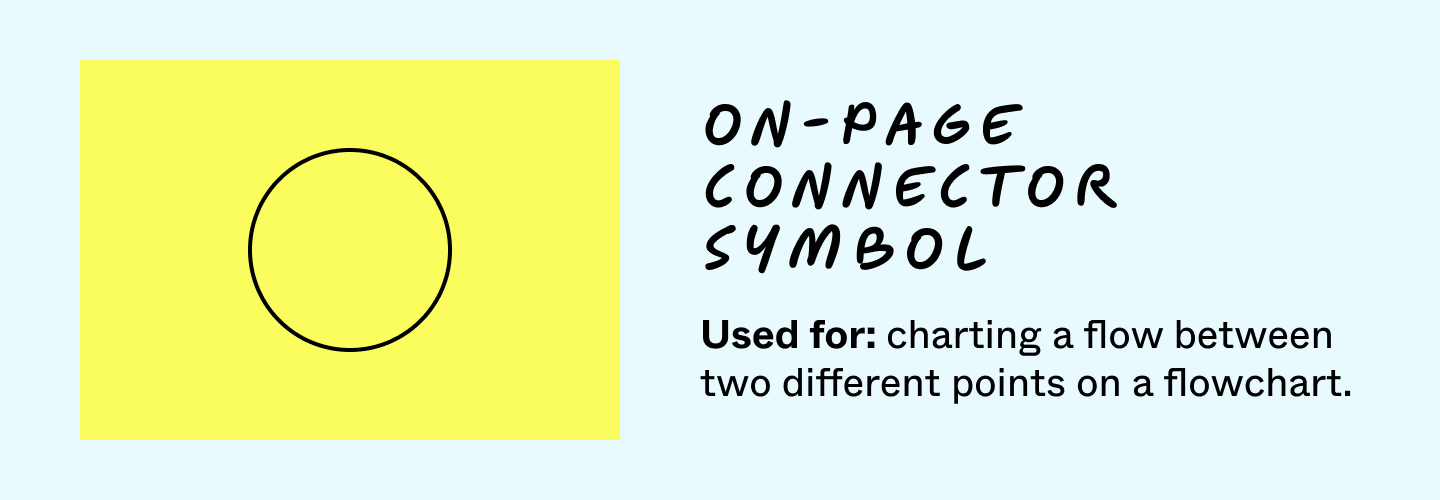
6.Connector line
Connector lines in flowcharts are used to show the direction of the flow of control from one symbol or step to another. They are crucial for indicating how different parts of the flowchart are related and how the process progresses.
Flow charts for Simple Problems
1.Write an algorithm and draw Flow chart to sum of three numbers
Algorithm
Start
- Begin the process.
Input Numbers
- Read the values of three numbers:
num1
num2
num3
Process
- Compute the sum of the three numbers: Sum=num1+num2+num3\text{Sum} = \text{num1} + \text{num2} + \text{num3}Sum=num1+num2+num3
Output Result
- Display the result of the sum.
End
- End the process.
Flowchart Representation

2.Write an algorithm and Flow Chart to find whether a given N number is positive or negative
Algorithm
- Start
- Input: Read the number NNN.
- Check:
- If N>0N > 0N>0, then NNN is positive.
- If N<0N < 0N<0, then NNN is negative.
- If N=0N = 0N=0, then NNN is neither positive nor negative.
- Output: Print whether NNN is positive, negative, or neither.
- End
