Introduction to Linked Lists in C
A linked list is a fundamental data structure that consists of a sequence of elements, where each element is connected to the next one through pointers. Unlike arrays, linked lists do not have a fixed size, allowing for dynamic memory allocation and efficient insertion and deletion of elements.
Key Concepts
- Node: Each element in a linked list is called a node. A node typically contains data and a pointer to the next node in the sequence.
- Head: The head is the first node of the linked list. It is used as a starting point for any operation on the list.
- Pointer: Pointers are used to connect nodes in the linked list, allowing traversal from one node to another.
Types of Linked Lists
- Singly Linked List: Each node points to the next node and the last node points to NULL.
- Doubly Linked List: Each node contains pointers to both the next and the previous nodes.
- Circular Linked List: The last node points back to the head, forming a circle.
Difference Between Linked list and Arrays:
Aspect | Array | Linked List |
---|---|---|
Memory Allocation | Contiguous memory allocation. | Non-contiguous memory allocation. |
Size | Fixed size determined at compile-time. | Dynamic size; can grow or shrink during runtime. |
Access Time | Constant time (O(1)O(1)) for accessing elements by index. | Linear time (O(n)O(n)) for accessing elements (sequential access). |
Insertion/Deletion | Inefficient for insertion/deletion in the middle (O(n)O(n)) due to shifting elements. | Efficient for insertion/deletion (O(1)O(1) if the node is known), requires pointer adjustment. |
Memory Usage | Requires less memory; no extra storage for pointers. | Requires more memory; each node stores an additional pointer. |
Traversal | Can be indexed directly for easy traversal. | Requires sequential traversal using pointers. |
Cache Performance | Better cache performance due to locality of reference. | Poor cache performance due to scattered nodes in memory. |
Data Storage | Suitable for static data storage. | Suitable for dynamic data storage. |
Multi-dimensional | Supports multi-dimensional arrays (e.g., 2D arrays). | Does not directly support multi-dimensional storage. |
Complexity | Simple to implement and use. | More complex due to pointer handling and dynamic memory management. |
Memory Reallocation | Difficult and costly; requires creating a new larger array. | Easy, as linked lists naturally accommodate growth. |
Application | Useful for applications where frequent access and fixed size are needed. | Useful for applications where frequent insertion/deletion and dynamic size are needed. |
Summary
- Arrays are suitable for scenarios where you need fast access to elements and the data size is known and fixed. They are efficient in terms of access time and memory usage, but they lack flexibility for dynamic resizing and frequent insertions/deletions.
- Linked Lists are ideal when you need a data structure that can dynamically change size and where insertions and deletions are frequent. They provide flexibility but at the cost of increased memory usage and slower access times.
Each structure has its own strengths and weaknesses, and the choice between arrays and linked lists depends on the specific requirements of the application.
Advantages and Disadvantages of Linked list:
Linked lists are a fundamental data structure used in computer science for dynamic storage of data. They have various advantages and disadvantages, which make them suitable for specific use cases. Below is a detailed breakdown of the advantages and disadvantages of linked lists.
Advantages of Linked Lists
- Dynamic Size:
- Description: Linked lists can grow and shrink dynamically as needed, which makes them suitable for applications where the number of elements is not known in advance.
- Benefit: Efficient memory utilization as there is no need to allocate a fixed amount of memory in advance.
- Efficient Insertions/Deletions:
- Description: Inserting or deleting a node in a linked list does not require shifting elements, as it does in arrays.
- Benefit: Operations can be done in constant time O(1)O(1)O(1) if the pointer to the previous node is known, making it ideal for scenarios where frequent insertions and deletions are needed.
- Flexible Memory Use:
- Description: Nodes are allocated as needed and do not need contiguous memory space.
- Benefit: Linked lists can utilize fragmented memory and handle dynamic allocation better than arrays.
- Ease of Implementation for Certain Data Structures:
- Description: Linked lists are the basis for implementing other complex data structures like stacks, queues, and graphs.
- Benefit: Simplifies the implementation of more complex structures due to inherent link-based structure.
- Efficient Data Rearrangement:
- Description: Data rearrangement is easier as only the pointers need to be adjusted.
- Benefit: Efficient for applications that require frequent reordering or rearrangement of elements.
Disadvantages of Linked Lists
- Increased Memory Usage:
- Description: Each node in a linked list requires additional memory for a pointer to the next node.
- Drawback: Higher memory overhead compared to arrays, especially in small data elements.
- Sequential Access:
- Description: Accessing elements requires traversal from the head node, leading to linear time complexity O(n)O(n)O(n).
- Drawback: Slower access times compared to arrays, which provide constant time access O(1)O(1)O(1) via indexing.
- Complexity in Implementation:
- Description: Implementing linked lists requires careful management of pointers and can be error-prone.
- Drawback: More complex to implement and manage than arrays, increasing the potential for bugs.
- Poor Cache Locality:
- Description: Linked lists have poor locality of reference as nodes are scattered across memory.
- Drawback: Decreased cache performance and slower access speeds compared to arrays, which have excellent cache performance due to contiguous memory allocation.
- Extra Operations for Backward Traversal:
- Description: Singly linked lists do not support backward traversal without additional pointers.
- Drawback: Doubly linked lists can solve this but require even more memory overhead for an extra pointer.
- Difficulty in Finding the Length:
- Description: Calculating the length of a linked list requires traversing all nodes.
- Drawback: Increased time complexity for operations that depend on knowing the length.
When to Use Linked Lists
Linked lists are ideal for applications where:
- The number of elements is unknown and can change frequently.
- Frequent insertions and deletions are required.
- Memory allocation should be flexible and non-contiguous.
When Not to Use Linked Lists
Linked lists might not be the best choice when:
- Fast random access to elements is required.
- Memory overhead is a critical concern.
- Cache performance is a significant consideration.
Conclusion
Linked lists provide flexibility and efficiency for dynamic data management but come with trade-offs in terms of memory usage and access speed. Understanding these advantages and disadvantages helps in choosing the right data structure based on specific application needs.
Purpose of Dummy Header
A dummy header (or sentinel node) in a linked list is an auxiliary node placed at the beginning of the list. It does not hold any meaningful data but serves as a placeholder to simplify certain list operations, such as insertion and deletion, by reducing the number of special cases that need to be handled.
Single linked list
A singly linked list is a fundamental data structure that consists of a sequence of nodes, where each node contains data and a pointer to the next node in the sequence. It is a simple yet powerful structure used to store and manage collections of data. Singly linked lists are widely used in computer science due to their dynamic nature and ease of insertion and deletion operations.
Structure of a Singly Linked List
A singly linked list is composed of nodes, each of which has two components:
- Data: The actual data or value that the node holds.
- Next Pointer: A pointer (or reference) to the next node in the list. The last node’s next pointer points to
NULL
, indicating the end of the list.
Here’s a simple representation:
Operations on Singly Linked Lists
1. Insertion
- At the Beginning: Insert a new node at the head of the list.
- At the End: Traverse to the end and insert the node after the last node.
- After a Given Node: Insert a node after a specified node.
2. Deletion
- From the Beginning: Remove the node at the head of the list.
- From the End: Traverse and remove the last node.
- After a Given Node: Remove a node after a specified node.
3. Traversal
- Visit each node starting from the head and proceed to the next node until reaching the end of the list.
4. Search
- Traverse the list to find a node containing a specified value.
5. Update
- Change the data in a node at a particular position.
Advantages of Singly Linked Lists
- Dynamic Size: Can grow or shrink as needed, allowing efficient memory use.
- Efficient Insertions/Deletions: Adding or removing nodes can be done without shifting elements.
- Simplicity: Easy to implement and manage for simple applications.
Disadvantages of Singly Linked Lists
- Sequential Access: Must traverse nodes sequentially to access elements.
- Increased Memory Usage: Requires extra memory for pointers in each node.
- Poor Cache Performance: Nodes may not be stored contiguously, leading to slower access.
Program for Creating Single Linked list
#include <stdio.h>
#include <stdlib.h>
// Define a node structure
typedef struct Node {
int data; // Data stored in the node
struct Node* next; // Pointer to the next node
} Node;
// Function to create a new node
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node)); // Allocate memory for the node
if (newNode == NULL) { // Check for memory allocation failure
printf("Memory allocation failed\n");
exit(1);
}
newNode->data = data; // Set the node's data
newNode->next = NULL; // Initialize the next pointer to NULL
return newNode;
}
// Function to create and initialize the linked list
Node* createLinkedList() {
// Create the head of the list
Node* head = createNode(10); // Create the first node with data 10
// Create more nodes
Node* second = createNode(20); // Create the second node with data 20
Node* third = createNode(30); // Create the third node with data 30
// Link the nodes
head->next = second; // Link the head to the second node
second->next = third; // Link the second node to the third node
return head; // Return the head of the linked list
}
// Function to print the linked list
void printList(Node* node) {
while (node != NULL) { // Traverse until the end of the list
printf("%d -> ", node->data);
node = node->next; // Move to the next node
}
printf("NULL\n");
}
// Main function to test the linked list creation
int main() {
Node* head = createLinkedList(); // Create the linked list
// Print the linked list
printf("Linked list: ");
printList(head);
return 0;
}
–>Click here for detail Explanation of Single Linked list program
1. Defining the Node Structure
In a singly linked list, each node contains two main components:
- Data: This is the value or information the node holds.
- Next Pointer: This is a pointer that points to the next node in the list. In the last node, this pointer is set to
NULL
to indicate the end of the list.
Here is how you define the structure for a node:
typedef struct Node {
int data; // Data stored in the node
struct Node* next; // Pointer to the next node
} Node;
2. Creating Nodes
To create a new node, you need to allocate memory for it, set its data, and initialize its next
pointer to NULL
. This is done using the createNode
function:
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node)); // Allocate memory for the node
if (newNode == NULL) { // Check if memory allocation failed
printf("Memory allocation failed\n");
exit(1); // Exit if memory allocation fails
}
newNode->data = data; // Set the node's data
newNode->next = NULL; // Initialize the next pointer to NULL
return newNode; // Return the new node
}
malloc(sizeof(Node))
: Allocates memory for a new node. The size of the node is determined bysizeof(Node)
.newNode->data = data
: Sets the data for the node.newNode->next = NULL
: Initializes the next pointer toNULL
, as this node will not yet link to any other node.
3. Linking Nodes
Once nodes are created, you need to link them to form a list. This involves setting the next
pointer of one node to point to another node.
Here’s a function to create and initialize a simple linked list with three nodes:
Node* createLinkedList() {
// Create the head of the list
Node* head = createNode(10); // Create the first node with data 10
// Create more nodes
Node* second = createNode(20); // Create the second node with data 20
Node* third = createNode(30); // Create the third node with data 30
// Link the nodes
head->next = second; // Link the head node to the second node
second->next = third; // Link the second node to the third node
return head; // Return the head of the linked list
}
Node* head = createNode(10);
: Creates the first node with data10
and assigns it tohead
.Node* second = createNode(20);
: Creates the second node with data20
.Node* third = createNode(30);
: Creates the third node with data30
.head->next = second;
: Sets thenext
pointer of thehead
node to point to thesecond
node.second->next = third;
: Sets thenext
pointer of thesecond
node to point to thethird
node.
4. Printing the List
To visualize the contents of the linked list, you need a function to traverse the list and print each node’s data.
Here’s a function to print the linked list:
void printList(Node* node) {
while (node != NULL) { // Traverse until the end of the list
printf("%d -> ", node->data); // Print the data of the current node
node = node->next; // Move to the next node
}
printf("NULL\n"); // Print NULL to indicate the end of the list
}
while (node != NULL)
: Iterates through the list untilnode
isNULL
.printf("%d -> ", node->data)
: Prints the data of the current node followed by->
.node = node->next
: Moves to the next node in the list.printf("NULL\n")
: PrintsNULL
to indicate the end of the list.
5. Main Function
The main
function initializes the linked list and prints it.
int main() {
Node* head = createLinkedList(); // Create the linked list
// Print the linked list
printf("Linked list: ");
printList(head);
return 0; // Return 0 to indicate successful completion
}
Node* head = createLinkedList();
: CallscreateLinkedList
to initialize the list and assigns the head of the list tohead
.printList(head);
: CallsprintList
to print the contents of the linked list.
Summary
- Define Node Structure: Each node has data and a pointer to the next node.
- Create Nodes: Allocate memory and initialize nodes.
- Link Nodes: Set the
next
pointer to connect nodes. - Print List: Traverse and print node data.
- Main Function: Initialize the list and print it.
Perform Insertion and Deletion operation on a Single Linked list
Singly Linked List Structure
A singly linked list is a series of nodes where each node contains:
- Data: The value in the node.
- Next: A pointer/reference to the next node in the list.
Here’s a visual representation:
Head -> [Data | Next] -> [Data | Next] -> [Data | Next] -> [Data | NULL]
Insertion Operation
Let’s say you want to insert a new node with data 5
after the node with data 2
.
Initial List:
Head -> [1 | *] -> [2 | *] -> [3 | *] -> [4 | NULL]
Step 1: Locate the Position for Insertion
Find the node with data 2
.
Step 2: Create the New Node
Create a new node with data 5
:
New Node -> [5 | *]
Step 3: Update Pointers
- Set the
Next
Pointer of the New Node: Point it to the node that comes after the node with data2
(which is the node with data3
). - Update the
Next
Pointer of the Node with Data2
: Point it to the new node.
Resulting List:
Head -> [1 | *] -> [2 | *] -> [5 | *] -> [3 | *] -> [4 | NULL]
Deletion Operation
Now, let’s delete the node with data 3
.
Initial List:
Head -> [1 | *] -> [2 | *] -> [5 | *] -> [3 | *] -> [4 | NULL]
Step 1: Locate the Node to Delete
Find the node with data 3
and its predecessor, which is the node with data 5
.
Step 2: Update Pointers
- Update the
Next
Pointer of the Node with Data5
: Point it to the node that comes after the node with data3
(which is the node with data4
). - Remove the Node: The node with data
3
is now bypassed and effectively removed from the list.
Resulting List:
Head -> [1 | *] -> [2 | *] -> [5 | *] -> [4 | NULL]
Summary
- Insertion:
- Find where to insert.
- Create the new node.
- Adjust pointers so that the new node is inserted in the list.
- Deletion:
- Find the node to delete and its predecessor.
- Adjust pointers to bypass the node to be deleted.
- The node is removed from the list.
Program for Inserting and Deleting nodes
#include <stdio.h>
#include <stdlib.h>
// Definition of a node
typedef struct Node {
int data;
struct Node* next;
} Node;
// Function to create a new node
Node* create_node(int data) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->data = data;
new_node->next = NULL;
return new_node;
}
// Function to insert a node at the beginning
void insert_at_beginning(Node** head_ref, int data) {
Node* new_node = create_node(data);
new_node->next = *head_ref;
*head_ref = new_node;
}
// Function to insert a node at the end
void insert_at_end(Node** head_ref, int data) {
Node* new_node = create_node(data);
if (*head_ref == NULL) {
*head_ref = new_node;
return;
}
Node* temp = *head_ref;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = new_node;
}
// Function to delete a node with a specific value
void delete_node(Node** head_ref, int key) {
Node* temp = *head_ref;
Node* prev = NULL;
// If the head node itself holds the key
if (temp != NULL && temp->data == key) {
*head_ref = temp->next; // Change head
free(temp); // Free old head
return;
}
// Search for the key to be deleted
while (temp != NULL && temp->data != key) {
prev = temp;
temp = temp->next;
}
// If the key was not present in the list
if (temp == NULL) return;
// Unlink the node from the linked list
prev->next = temp->next;
free(temp); // Free the memory
}
// Function to print the linked list
void print_list(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL;
// Inserting elements at the beginning
insert_at_beginning(&head, 10);
insert_at_beginning(&head, 20);
insert_at_beginning(&head, 30);
// Inserting elements at the end
insert_at_end(&head, 40);
insert_at_end(&head, 50);
printf("Linked list before deletion:\n");
print_list(head);
// Deleting a node
delete_node(&head, 20);
printf("Linked list after deletion:\n");
print_list(head);
return 0;
}
–>Click here for detail Explanation of Insertion and Deleting nodes in single linked list program
Demonstrates insertion at the beginning and end, prints the list before and after deleting a node, and finally prints the updated list.
Node Definition:
typedef struct Node
defines a node in the linked list with an int
data field and a next
pointer to the next node.
Creating a New Node:
create_node(int data)
allocates memory for a new node, initializes its data, and sets its next
pointer to NULL
.
Insertion at the Beginning:
insert_at_beginning(Node** head_ref, int data)
creates a new node and inserts it at the start of the linked list. The head_ref
is updated to point to the new node.
Insertion at the End:
insert_at_end(Node** head_ref, int data)
creates a new node and inserts it at the end of the linked list. It traverses to the last node and then links the new node.
Deletion of a Node:
delete_node(Node** head_ref, int key)
deletes the first occurrence of a node with the specified key. It handles the special case where the node to be deleted is the head of the list.
Printing the Linked List:
print_list(Node* head)
traverses the linked list and prints each node’s data, ending with NULL
.
Main Function:
Structure of a Single Circular Linked list
A Single Circular Linked List (SCLL) is a type of linked list where each node points to the next node in the sequence, and the last node points back to the first node, forming a circular structure. This means there is no null
reference to signify the end of the list.
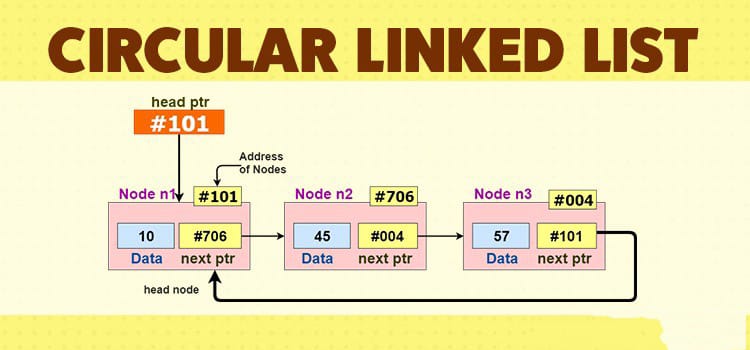
Here’s a breakdown of its key features and components:
- Nodes: Each node in a single circular linked list contains two parts:
- Data: The value or information stored in the node.
- Next Pointer: A reference to the next node in the list.
- Circular Structure: Unlike a linear linked list where the last node points to
null
, in a circular linked list, the last node’sNext Pointer
refers back to the first node. This creates a circular chain of nodes. - Head Node: The list has a
head
node that acts as the starting point of the list. From the head, you can traverse the entire list by following theNext Pointers
until you reach the starting node again.
Program for single circular linked list
#include <stdio.h>
#include <stdlib.h>
// Node structure
typedef struct Node {
int data;
struct Node* next;
} Node;
// Function to create a new node
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = newNode; // Initialize next to point to itself
return newNode;
}
// Function to insert a node at the end
void insertEnd(Node** head, int data) {
Node* newNode = createNode(data);
if (*head == NULL) {
*head = newNode;
return;
}
Node* temp = *head;
while (temp->next != *head) {
temp = temp->next;
}
temp->next = newNode;
newNode->next = *head;
}
// Function to delete a node
void deleteNode(Node** head, int key) {
if (*head == NULL) {
return;
}
Node *temp = *head, *prev = NULL;
// If the node to be deleted is the head node
if (temp->data == key) {
prev = *head;
while (prev->next != *head) {
prev = prev->next;
}
if (*head == (*head)->next) {
free(*head);
*head = NULL;
} else {
prev->next = (*head)->next;
free(*head);
*head = prev->next;
}
return;
}
// Search for the node to be deleted
while (temp->next != *head && temp->next->data != key) {
temp = temp->next;
}
// Node not found
if (temp->next == *head) {
return;
}
// Node found
Node* nodeToDelete = temp->next;
temp->next = nodeToDelete->next;
free(nodeToDelete);
}
// Function to traverse and print the list
void printList(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = head;
do {
printf("%d -> ", temp->data);
temp = temp->next;
} while (temp != head);
printf("(head)\n");
}
// Main function
int main() {
Node* head = NULL;
insertEnd(&head, 1);
insertEnd(&head, 2);
insertEnd(&head, 3);
insertEnd(&head, 4);
printf("Circular Linked List:\n");
printList(head);
printf("Deleting node with data 2\n");
deleteNode(&head, 2);
printf("Circular Linked List after deletion:\n");
printList(head);
return 0;
}
–>Click here for detail Explanation of Single circular linked list program
1. Node Structure
typedef struct Node {
int data;
struct Node* next;
} Node;
- Node Structure: This defines a
Node
which has two parts:data
: Stores the value (in this case, an integer).next
: A pointer to the next node in the list. Since this is a circular linked list, thenext
pointer will eventually point back to the head node or another node, forming a loop.
2. Create a New Node
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = newNode; // Initialize next to point to itself
return newNode;
}
- Function Purpose: Creates and initializes a new node.
- Details:
malloc(sizeof(Node))
: Allocates memory for a new node.newNode->data = data
: Sets the data of the new node.newNode->next = newNode
: Initially, thenext
pointer of a single node points to itself. This is because if this is the only node in the list, it forms a circular link to itself.
3. Insert a Node at the End
void insertEnd(Node** head, int data) {
Node* newNode = createNode(data);
if (*head == NULL) {
*head = newNode;
return;
}
Node* temp = *head;
while (temp->next != *head) {
temp = temp->next;
}
temp->next = newNode;
newNode->next = *head;
}
- Function Purpose: Inserts a new node at the end of the list.
- Details:
Node* newNode = createNode(data)
: Creates a new node with the provided data.if (*head == NULL)
: Checks if the list is empty (i.e., no head node). If it is, the new node becomes the head.while (temp->next != *head)
: Traverses the list to find the node that currently points back to the head.temp->next = newNode
: Updates thenext
pointer of the last node to point to the new node.newNode->next = *head
: The new node’snext
pointer should point back to the head node to maintain the circular nature.
4. Delete a Node
void deleteNode(Node** head, int key) {
if (*head == NULL) {
return;
}
Node *temp = *head, *prev = NULL;
if (temp->data == key) {
prev = *head;
while (prev->next != *head) {
prev = prev->next;
}
if (*head == (*head)->next) {
free(*head);
*head = NULL;
} else {
prev->next = (*head)->next;
free(*head);
*head = prev->next;
}
return;
}
while (temp->next != *head && temp->next->data != key) {
temp = temp->next;
}
if (temp->next == *head) {
return;
}
Node* nodeToDelete = temp->next;
temp->next = nodeToDelete->next;
free(nodeToDelete);
}
- Function Purpose: Deletes the first node with the specified
data
. - Details:
if (*head == NULL)
: Checks if the list is empty. If so, there’s nothing to delete.if (temp->data == key)
: Checks if the node to be deleted is the head node.- If the head node is the only node, it is deleted, and the list is set to
NULL
. - Otherwise, find the last node (which points to the head) and adjust its
next
pointer to skip the head node.
- If the head node is the only node, it is deleted, and the list is set to
while (temp->next != *head && temp->next->data != key)
: Searches for the node with the specified data.Node* nodeToDelete = temp->next
: If found, adjust pointers to remove the node from the list and free its memory.
5. Print the List
void printList(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = head;
do {
printf("%d -> ", temp->data);
temp = temp->next;
} while (temp != head);
printf("(head)\n");
}
- Function Purpose: Prints the contents of the list.
- Details:
if (head == NULL)
: Checks if the list is empty.do { ... } while (temp != head)
: Traverses the list starting from the head and prints each node’s data. The loop continues until it returns to the head node, ensuring that the entire list is printed.
6. Main Function
int main() {
Node* head = NULL;
insertEnd(&head, 1);
insertEnd(&head, 2);
insertEnd(&head, 3);
insertEnd(&head, 4);
printf("Circular Linked List:\n");
printList(head);
printf("Deleting node with data 2\n");
deleteNode(&head, 2);
printf("Circular Linked List after deletion:\n");
printList(head);
return 0;
}
Purpose: Demonstrates how to use the list functions.
Details:
Initializes an empty list (head = NULL
).
Inserts four nodes with data 1, 2, 3, 4
.
Prints the list.
Deletes the node with data 2
.
Prints the list again after deletion.
Double Linked list
A doubly linked list is a data structure that consists of a set of nodes, each of which contains a value and two pointers, one pointing to the previous node in the list and one pointing to the next node in the list. This allows for efficient traversal of the list in both directions, making it suitable for applications where frequent insertions and deletions are required.
Representation of Doubly Linked List in Data Structure:
In a data structure, a doubly linked list is represented using nodes that have three fields:
- Data
- A pointer to the next node (next)
- A pointer to the previous node (prev).
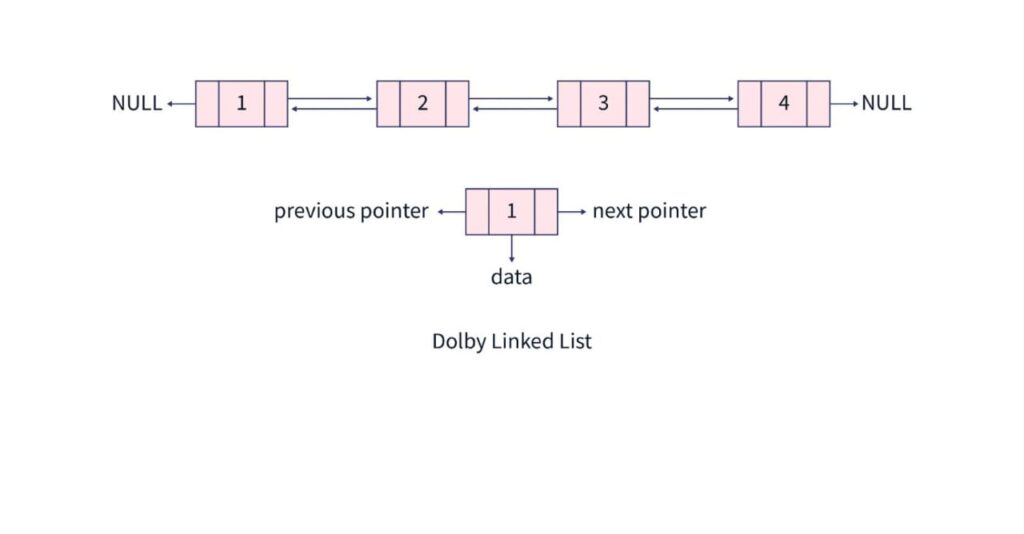
Insertion at the Beginning in Doubly Linked List:
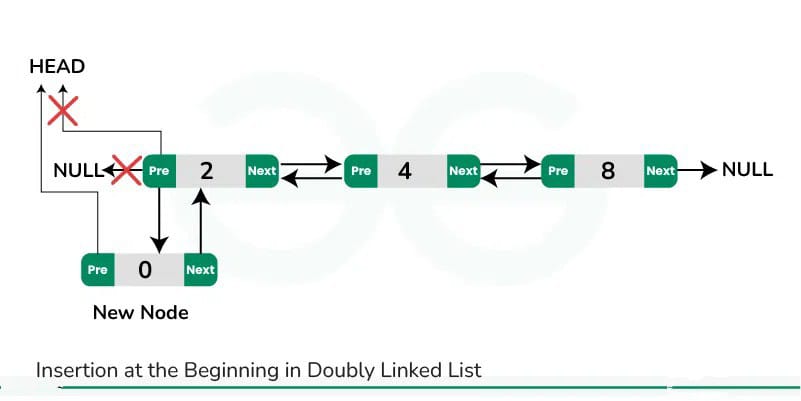
To insert a new node at the beginning of a doubly linked list, follow these steps:
- Allocate Memory: Create a new node and assign the provided value to its
data
field. - Initialize Pointers:
- Set the
prev
pointer of the new node toNULL
.
- Set the
- Check if the List is Empty:
- If the list is empty (
head
isNULL
):- Set the
next
pointer of the new node toNULL
. - Update the
head
pointer to point to the new node.
- Set the
- If the list is empty (
- If the List is Not Empty:
- Set the
next
pointer of the new node to the current head node. - Update the
prev
pointer of the current head node to point to the new node. - Update the
head
pointer to point to the new node.
- Set the
Insertion at the End of Doubly Linked List
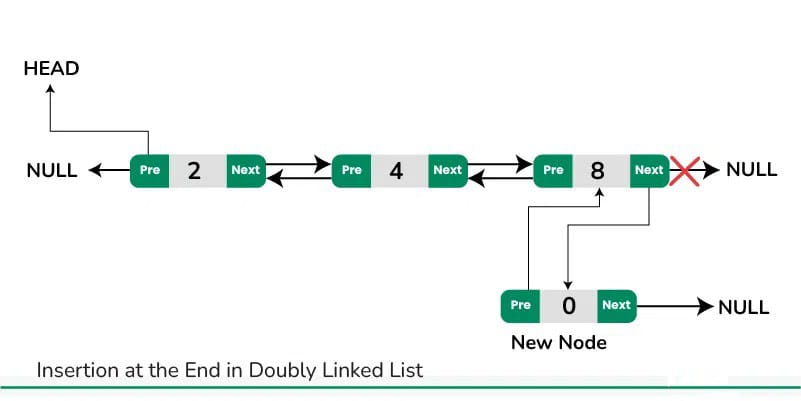
To insert a new node at the end of the doubly linked list, we can use the following steps:
- Allocate memory for a new node and assign the provided value to its data field.
- Initialize the next pointer of the new node to nullptr.
- If the list is empty:
- Set the previous pointer of the new node to nullptr.
- Update the head pointer to point to the new node.
- If the list is not empty:
- Traverse the list starting from the head to reach the last node.
- Adjust pointers to insert the new node at the end:
- Set the next pointer of the last node to point to the new node.
- Set the previous pointer of the new node to point to the last node.’
Insertion at a Specific Position of Doubly Linked List
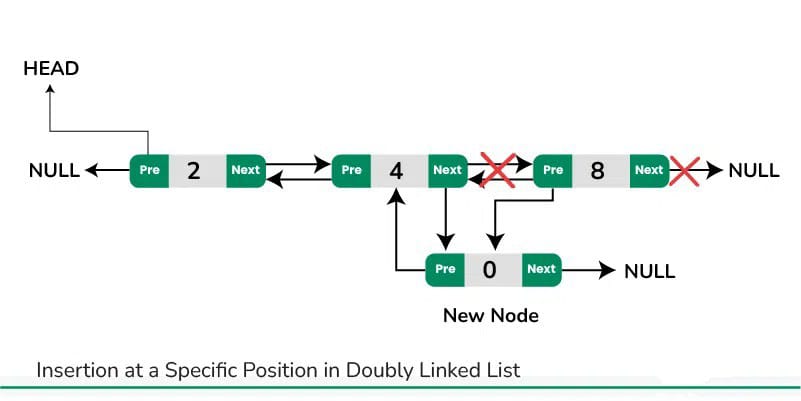
To insert a node at a specific Position in doubly linked list, we can use the following steps:
- Traverse the list to find the node at the desired position.
- Create a new node with the data you want to insert.
- Adjust the pointers of the new node, the previous node, and the next node to include the new node in the list.
- Update the pointers of the neighboring nodes to maintain the doubly linked list structure.
Deletion at the Beginning of doubly linked list
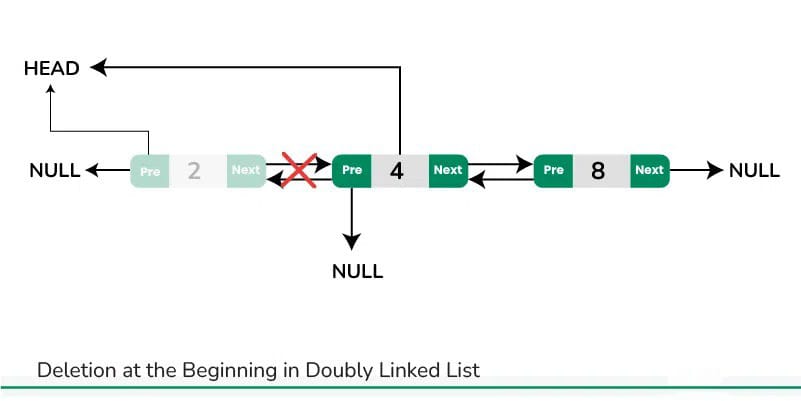
To delete a node at the beginning of a doubly linked list, follow these steps:
- Check if the List is Empty:
- If the list is empty (
head
isNULL
), there is nothing to delete. Return immediately.
- If the list is empty (
- Delete the Head Node:
- If the node to be deleted is the head node:
- Update the
head
pointer to point to the next node in the list. - If the new head node is not
NULL
, update itsprev
pointer toNULL
.
- Update the
- If the node to be deleted is the head node:
- Free Memory:
- Deallocate the memory used by the node to be deleted.
Deletion at the End on doubly linked list:
To delete a node at the end of a doubly linked list, follow these steps:
- Check if the List is Empty:
- If the list is empty (
head
isNULL
), there is nothing to delete. Return from the function.
- If the list is empty (
- Find the Last Node:
- Traverse the list to reach the last node (the node whose
next
pointer isNULL
).
- Traverse the list to reach the last node (the node whose
- Update the Second-to-Last Node:
- If the list has more than one node, set the
next
pointer of the second-to-last node toNULL
. This makes it the new last node.
- If the list has more than one node, set the
- Free Memory:
- Free the memory allocated for the node that was deleted (the original last node).
Deletion at a Specific Position in doubly linked list:
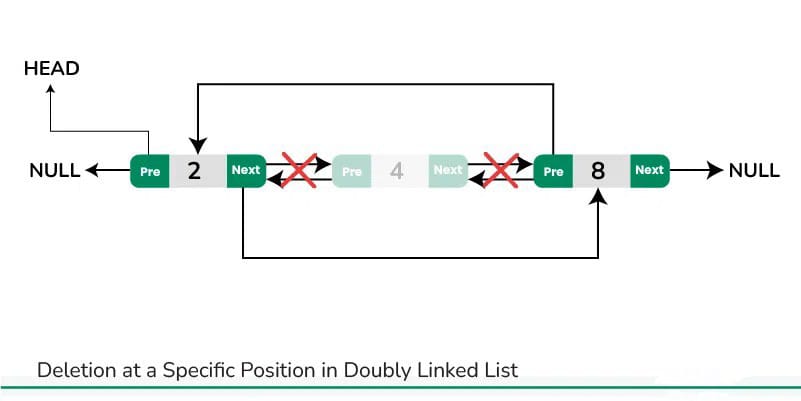
To delete a node at a specific position in a doubly linked list, follow these steps:
- Traverse to the Node at the Specified Position:
- Start from the head and move through the list to reach the node at the given position. Ensure you handle edge cases, such as positions that are out of bounds.
- Adjust Pointers:
- If Deleting the Head Node:
- Update the
head
pointer to the next node. - If the new head is not
NULL
, set itsprev
pointer toNULL
.
- Update the
- If Deleting a Node Other Than the Head:
- Update the
next
pointer of the node before the deleted node to point to the node after the deleted node. - Update the
prev
pointer of the node after the deleted node to point to the node before the deleted node.
- Update the
- If Deleting the Head Node:
- Free Memory:
- Release the memory allocated for the deleted node.
Program for Double linked list :
#include <stdio.h>
#include <stdlib.h>
// Node structure
typedef struct Node {
int data;
struct Node* next;
struct Node* prev;
} Node;
// Function to create a new node
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
newNode->prev = NULL;
return newNode;
}
// Function to insert a node at the beginning
void insertFront(Node** head, int data) {
Node* newNode = createNode(data);
newNode->next = *head;
if (*head != NULL) {
(*head)->prev = newNode;
}
*head = newNode;
}
// Function to insert a node at the end
void insertEnd(Node** head, int data) {
Node* newNode = createNode(data);
Node* last = *head;
if (*head == NULL) {
*head = newNode;
return;
}
while (last->next != NULL) {
last = last->next;
}
last->next = newNode;
newNode->prev = last;
}
// Function to insert a node after a specific node
void insertAfter(Node* prevNode, int data) {
if (prevNode == NULL) return;
Node* newNode = createNode(data);
newNode->next = prevNode->next;
newNode->prev = prevNode;
if (prevNode->next != NULL) {
prevNode->next->prev = newNode;
}
prevNode->next = newNode;
}
// Function to delete a node at a specific position
void deleteNode(Node** head, int position) {
if (*head == NULL) return;
Node* temp = *head;
// If head node needs to be removed
if (position == 0) {
*head = temp->next;
if (*head != NULL) {
(*head)->prev = NULL;
}
free(temp);
return;
}
// Traverse to the node to be deleted
for (int i = 0; temp != NULL && i < position; i++) {
temp = temp->next;
}
// Node not found
if (temp == NULL) return;
// Adjust pointers
if (temp->next != NULL) {
temp->next->prev = temp->prev;
}
if (temp->prev != NULL) {
temp->prev->next = temp->next;
}
free(temp);
}
// Function to print the list from head to end
void printListForward(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d <-> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
// Function to print the list from end to head
void printListBackward(Node* head) {
if (head == NULL) return;
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
while (temp != NULL) {
printf("%d <-> ", temp->data);
temp = temp->prev;
}
printf("NULL\n");
}
// Main function to demonstrate the use of the list
int main() {
Node* head = NULL;
// Insert elements
insertFront(&head, 1);
insertEnd(&head, 2);
insertEnd(&head, 3);
insertAfter(head, 4); // Inserting after the head node
printf("Doubly Linked List (Forward):\n");
printListForward(head);
printf("Doubly Linked List (Backward):\n");
printListBackward(head);
printf("Deleting node at position 2\n");
deleteNode(&head, 2);
printf("Doubly Linked List after deletion (Forward):\n");
printListForward(head);
printf("Doubly Linked List after deletion (Backward):\n");
printListBackward(head);
return 0;
}
–>Click here for detail Explanation for double linked list program
1. Node Structure
typedef struct Node {
int data; // Holds the value of the node
struct Node* next; // Pointer to the next node in the list
struct Node* prev; // Pointer to the previous node in the list
} Node;
data
: Stores the value of the node. This can be any type of data; in this case, it’s an integer.next
: Points to the next node in the list. If it’s the last node, it points toNULL
.prev
: Points to the previous node in the list. If it’s the first node, it points toNULL
.
2. Creating a New Node
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node)); // Allocate memory for a new node
newNode->data = data; // Set the data field
newNode->next = NULL; // Initialize the next pointer to NULL
newNode->prev = NULL; // Initialize the prev pointer to NULL
return newNode;
}
malloc(sizeof(Node))
: Allocates memory for a new node. Thesizeof(Node)
ensures enough space for all parts of the node.newNode->data = data
: Assigns the value provided as an argument to thedata
field of the new node.newNode->next = NULL
andnewNode->prev = NULL
: Initializes the pointers toNULL
because this node will not yet be linked to any other node.
3. Inserting at the Beginning
void insertFront(Node** head, int data) {
Node* newNode = createNode(data); // Create a new node
newNode->next = *head; // Point the new node’s next to the current head
if (*head != NULL) {
(*head)->prev = newNode; // Update the previous pointer of the current head
}
*head = newNode; // Update head to point to the new node
}
newNode->next = *head
: The new node’snext
pointer is set to the current head of the list. This means the new node will come before the current head.if (*head != NULL) { (*head)->prev = newNode; }
: If the list is not empty, update theprev
pointer of the current head to point to the new node.*head = newNode
: Update thehead
pointer to the new node. The new node is now the head of the list.
4. Inserting at the End
void insertEnd(Node** head, int data) {
Node* newNode = createNode(data); // Create a new node
Node* last = *head; // Start from the head to find the end
if (*head == NULL) {
*head = newNode; // If the list is empty, the new node is the head
return;
}
while (last->next != NULL) {
last = last->next; // Traverse to the last node
}
last->next = newNode; // Set the next pointer of the last node to the new node
newNode->prev = last; // Set the previous pointer of the new node to the last node
}
Node* last = *head
: Starts traversal from the head.while (last->next != NULL)
: Traverse until you reach the last node (wherenext
isNULL
).last->next = newNode
: Update thenext
pointer of the last node to the new node.newNode->prev = last
: Set theprev
pointer of the new node to the last node, linking it backward.
5. Inserting After a Specific Node
void insertAfter(Node* prevNode, int data) {
if (prevNode == NULL) return; // Ensure the previous node is not NULL
Node* newNode = createNode(data); // Create a new node
newNode->next = prevNode->next; // Set the new node’s next to the next of prevNode
newNode->prev = prevNode; // Set the new node’s previous to prevNode
if (prevNode->next != NULL) {
prevNode->next->prev = newNode; // Update the previous pointer of the node after prevNode
}
prevNode->next = newNode; // Set the next pointer of prevNode to the new node
}
newNode->next = prevNode->next
: The new node’snext
pointer is set to the node that followsprevNode
.newNode->prev = prevNode
: The new node’sprev
pointer is set toprevNode
.if (prevNode->next != NULL) { prevNode->next->prev = newNode; }
: Update theprev
pointer of the node that follows the new node.prevNode->next = newNode
: Set thenext
pointer ofprevNode
to the new node.
6. Deleting a Node at a Specific Position
void deleteNode(Node** head, int position) {
if (*head == NULL) return; // Ensure the list is not empty
Node* temp = *head;
// If the head node needs to be removed
if (position == 0) {
*head = temp->next; // Update the head to the next node
if (*head != NULL) {
(*head)->prev = NULL; // Update the prev pointer of the new head
}
free(temp); // Free the old head node
return;
}
// Traverse to the node to be deleted
for (int i = 0; temp != NULL && i < position; i++) {
temp = temp->next;
}
// Node not found
if (temp == NULL) return;
// Adjust pointers
if (temp->next != NULL) {
temp->next->prev = temp->prev; // Update the previous pointer of the node after temp
}
if (temp->prev != NULL) {
temp->prev->next = temp->next; // Update the next pointer of the node before temp
}
free(temp); // Free the memory allocated for the node to be deleted
}
if (*head == NULL) return;
: If the list is empty, there’s nothing to delete.if (position == 0)
: Special case for deleting the head node.*head = temp->next
: Update the head pointer to the next node.if (*head != NULL) { (*head)->prev = NULL; }
: If the new head exists, update itsprev
pointer.for (int i = 0; temp != NULL && i < position; i++)
: Traverse to the node at the specified position.if (temp == NULL) return;
: If the node is not found (position out of range), return.if (temp->next != NULL) { temp->next->prev = temp->prev; }
: Update theprev
pointer of the node aftertemp
.if (temp->prev != NULL) { temp->prev->next = temp->next; }
: Update thenext
pointer of the node beforetemp
.free(temp);
: Deallocate memory for the node.
7. Printing the List
Print Forward
void printListForward(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d <-> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
while (temp != NULL)
: Traverse from the head to the end of the list.printf("%d <-> ", temp->data)
: Print the data of the current node.temp = temp->next
: Move to the next node.printf("NULL\n")
: Indicate the end of the list.
Print Backward
void printListBackward(Node* head) {
if (head == NULL) return;
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
while (temp != NULL) {
printf("%d <-> ", temp->data);
temp = temp->prev;
}
printf("NULL\n");
}
while (temp->next != NULL)
: Traverse to the end of the list.while (temp != NULL)
: Traverse backward from the end to the beginning.printf("%d <-> ", temp->data)
: Print the data of the current node.
Double circular linked list
A double circular linked list is a variation of a doubly linked list where the list is circular. This means that the last node in the list points back to the first node, and the first node’s prev
pointer points to the last node. This structure allows traversal from any node in either direction and ensures that you can traverse the entire list starting from any node without needing to check for null pointers.
Definition of a Double Circular Linked List
In a double circular linked list, each node contains:
- Data Field: Holds the value or data of the node.
- Next Pointer: Points to the next node in the list.
- Previous Pointer: Points to the previous node in the list.
Characteristics:
- Circular: The
next
pointer of the last node points back to the first node, and theprev
pointer of the first node points back to the last node. - Doubly Linked: Each node has pointers to both its next and previous nodes, enabling bidirectional traversal.

Example program for Double Circular Linked list
#include <stdio.h>
#include <stdlib.h>
// Define the node structure
typedef struct Node {
int data;
struct Node* next;
struct Node* prev;
} Node;
// Function to create a new node
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = newNode;
newNode->prev = newNode;
return newNode;
}
// Function to insert a node at the end
void insertEnd(Node** head, int data) {
Node* newNode = createNode(data);
if (*head == NULL) {
*head = newNode;
} else {
Node* last = (*head)->prev;
last->next = newNode;
newNode->prev = last;
newNode->next = *head;
(*head)->prev = newNode;
}
}
// Function to insert a node at the beginning
void insertFront(Node** head, int data) {
Node* newNode = createNode(data);
if (*head == NULL) {
*head = newNode;
} else {
Node* last = (*head)->prev;
newNode->next = *head;
newNode->prev = last;
(*head)->prev = newNode;
last->next = newNode;
*head = newNode;
}
}
// Function to delete a node by value
void deleteNode(Node** head, int value) {
if (*head == NULL) return;
Node* temp = *head;
do {
if (temp->data == value) {
// Node to delete is the only node in the list
if (temp->next == temp) {
free(temp);
*head = NULL;
return;
}
// Node to delete is the head node
if (temp == *head) {
Node* last = (*head)->prev;
*head = (*head)->next;
(*head)->prev = last;
last->next = *head;
free(temp);
return;
}
// Node to delete is not the head node
temp->prev->next = temp->next;
temp->next->prev = temp->prev;
free(temp);
return;
}
temp = temp->next;
} while (temp != *head);
}
// Function to print the list forward
void printListForward(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = head;
do {
printf("%d <-> ", temp->data);
temp = temp->next;
} while (temp != head);
printf("HEAD\n");
}
// Function to print the list backward
void printListBackward(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = head->prev;
do {
printf("%d <-> ", temp->data);
temp = temp->prev;
} while (temp->next != head->prev);
printf("HEAD\n");
}
// Main function to demonstrate the double circular linked list
int main() {
Node* head = NULL;
// Insert elements
insertEnd(&head, 1);
insertEnd(&head, 2);
insertEnd(&head, 3);
insertFront(&head, 0);
printf("Doubly Circular Linked List (Forward):\n");
printListForward(head);
printf("Doubly Circular Linked List (Backward):\n");
printListBackward(head);
printf("Deleting node with value 2\n");
deleteNode(&head, 2);
printf("Doubly Circular Linked List after deletion (Forward):\n");
printListForward(head);
printf("Doubly Circular Linked List after deletion (Backward):\n");
printListBackward(head);
return 0;
}
–>Click here for detail explanation of Double Circular linked List Program
1. Node Structure
typedef struct Node {
int data; // Data stored in the node
struct Node* next; // Pointer to the next node in the list
struct Node* prev; // Pointer to the previous node in the list
} Node;
Explanation:
data
: Stores the value or data of the node. In this case, it’s an integer, but it can be any type.next
: Points to the next node in the list. For circular lists, this pointer of the last node will point back to the head.prev
: Points to the previous node in the list. For circular lists, this pointer of the head node will point to the last node.
2. Creating a Node
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node)); // Allocate memory for a new node
newNode->data = data; // Assign the data to the new node
newNode->next = newNode; // Initialize the next pointer to point to itself
newNode->prev = newNode; // Initialize the prev pointer to point to itself
return newNode;
}
Explanation:
malloc(sizeof(Node))
: Allocates memory for a new node. This memory will hold thedata
,next
, andprev
pointers.newNode->data = data
: Sets thedata
field of the new node.newNode->next = newNode
andnewNode->prev = newNode
: Initializes thenext
andprev
pointers to point to the node itself. This is important because when the list contains only this node, it should point to itself, maintaining circularity.
3. Inserting at the End
void insertEnd(Node** head, int data) {
Node* newNode = createNode(data); // Create a new node
if (*head == NULL) {
*head = newNode; // If the list is empty, make newNode the head
} else {
Node* last = (*head)->prev; // Find the last node in the list
last->next = newNode; // Set the next of the last node to newNode
newNode->prev = last; // Set the previous of newNode to the last node
newNode->next = *head; // Set the next of newNode to the head (circular connection)
(*head)->prev = newNode; // Update the head's previous to newNode
}
}
Explanation:
if (*head == NULL)
: Checks if the list is empty. If it is, the new node becomes the head of the list.Node* last = (*head)->prev
: Finds the last node in the list (i.e., the node whosenext
points to the head).last->next = newNode
: Links the last node to the new node.newNode->prev = last
: Links the new node back to the last node.newNode->next = *head
: Links the new node to the head, maintaining the circular nature.(*head)->prev = newNode
: Updates the head’sprev
pointer to point to the new node.
4. Inserting at the Beginning
void insertFront(Node** head, int data) {
Node* newNode = createNode(data); // Create a new node
if (*head == NULL) {
*head = newNode; // If the list is empty, make newNode the head
} else {
Node* last = (*head)->prev; // Find the last node in the list
newNode->next = *head; // Set the new node's next to the current head
newNode->prev = last; // Set the new node's prev to the last node
(*head)->prev = newNode; // Update the current head's prev to the new node
last->next = newNode; // Update the last node's next to the new node
*head = newNode; // Update the head to be the new node
}
}
Explanation:
if (*head == NULL)
: Checks if the list is empty. If so, the new node becomes the head.newNode->next = *head
: Sets the new node’snext
pointer to the current head.newNode->prev = last
: Sets the new node’sprev
pointer to the last node.(*head)->prev = newNode
: Updates the current head’sprev
pointer to the new node.last->next = newNode
: Updates the last node’snext
pointer to the new node.*head = newNode
: Updates the head to be the new node.
5. Deleting a Node
void deleteNode(Node** head, int value) {
if (*head == NULL) return; // Return if the list is empty
Node* temp = *head;
do {
if (temp->data == value) {
// Node to delete is the only node in the list
if (temp->next == temp) {
free(temp);
*head = NULL;
return;
}
// Node to delete is the head node
if (temp == *head) {
Node* last = (*head)->prev;
*head = (*head)->next;
(*head)->prev = last;
last->next = *head;
free(temp);
return;
}
// Node to delete is not the head node
temp->prev->next = temp->next;
temp->next->prev = temp->prev;
free(temp);
return;
}
temp = temp->next;
} while (temp != *head);
}
Explanation:
if (*head == NULL)
: Checks if the list is empty.do { ... } while (temp != *head);
: Traverses the list starting from the head and checks each node.if (temp->data == value)
: Checks if the current node contains the value to be deleted.if (temp->next == temp)
: Checks if the node to delete is the only node in the list.if (temp == *head)
: Handles the case where the node to delete is the head. Updates the head to the next node and adjusts pointers accordingly.temp->prev->next = temp->next
andtemp->next->prev = temp->prev
: Adjusts pointers to skip the node being deleted.
6. Printing the List Forward
void printListForward(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = head;
do {
printf("%d <-> ", temp->data);
temp = temp->next;
} while (temp != head);
printf("HEAD\n");
}
Explanation:
do { ... } while (temp != head);
: Traverses the list starting from the head and prints each node’s data until it loops back to the head.printf("%d <-> ", temp->data)
: Prints the data of each node.
7. Printing the List Backward
void printListBackward(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = head->prev;
do {
printf("%d <-> ", temp->data);
temp = temp->prev;
} while (temp->next != head->prev);
printf("HEAD\n");
}
Explanation:
Node* temp = head->prev;
: Starts traversal from the last node.do { ... } while (temp->next != head->prev);
: Traverses backward from the last node to the node before the head.printf("%d <-> ", temp->data)
: Prints the data of each node.
8. Main Function
int main() {
Node* head = NULL; // Initialize the head to NULL
// Insert elements
insertEnd(&head, 1);
insertEnd(&head, 2);
insertEnd(&head, 3);
insertFront(&head, 0);
// Print list forward and backward
printf("Doubly Circular Linked List (Forward):\n");
printListForward(head);
printf("Doubly Circular Linked List (Backward):\n");
printListBackward(head);
// Delete a node and print list again
printf("Deleting node with value 2\n");
deleteNode(&head, 2);
printf("Doubly Circular Linked List after deletion (Forward):\n");
printListForward(head);
printf("Doubly Circular Linked List after deletion (Backward):\n");
printListBackward(head);
return 0;
}
Explanation:
Node* head = NULL;
: Initializes the head pointer toNULL
, indicating an empty list.insertEnd(&head, 1);
: Inserts nodes at the end of the list.insertFront(&head, 0);
: Inserts a node at the beginning of the list.printListForward(head);
andprintListBackward(head);
: Print the list in both directions.deleteNode(&head, 2);
: Deletes a node with the value2
and prints the list again.
This program demonstrates the creation, insertion, deletion, and traversal of a double circular linked list. The use of circular pointers ensures that the list can be traversed starting from any node and will eventually loop back to the starting node.
Advantages and disadvantages of a single circular linked list over a singly linked list
Single Circular Linked List
Advantages:
- Circular Traversal:
- You can traverse the list starting from any node and eventually return to the starting node. This is useful for applications requiring circular traversal, such as round-robin scheduling.
- No Need to Check for End:
- You don’t need to handle the end of the list explicitly, as the last node’s
next
pointer points back to the head. This can simplify some operations, especially when performing cyclic operations.
- You don’t need to handle the end of the list explicitly, as the last node’s
- Efficient for Certain Operations:
- Operations like traversing from any node to another node in a circular fashion are straightforward. For example, finding the next node after the current one is simple, without needing to check if the end has been reached.
Disadvantages:
- Complexity in Insertion/Deletion:
- While insertion and deletion operations are possible, they can be more complex to implement than in a singly linked list. Care must be taken to correctly update the circular links.
- Potential Infinite Loops:
- In certain scenarios, if not handled properly, circular lists might cause infinite loops during traversal. This is because the list continuously loops back to the head.
- Difficulty in Traversing Backwards:
- Unlike doubly circular linked lists, single circular linked lists do not support backward traversal. If you need to traverse the list in both directions, you need a different structure.
Singly Linked List
Advantages:
- Simplicity:
- Singly linked lists are simpler to implement compared to circular linked lists. Each node has a single pointer to the next node, and there’s no need to manage circular links.
- Ease of Use:
- Operations like insertion, deletion, and traversal are easier to understand and implement. The end of the list is explicitly marked by a
NULL
pointer.
- Operations like insertion, deletion, and traversal are easier to understand and implement. The end of the list is explicitly marked by a
- Good for Linear Traversal:
- Ideal for applications where you need to process the list in a linear fashion, where each node is visited only once.
Disadvantages:
- End of List Check:
- You need to handle the end of the list explicitly (i.e., checking for
NULL
), which adds a small amount of complexity to traversal and certain operations.
- You need to handle the end of the list explicitly (i.e., checking for
- No Circular Traversal:
- Traversing from the end to the beginning is not possible. This can be a limitation for certain use cases where circular traversal is needed.
- Wasted Memory:
- Sometimes, the linear nature can lead to wasted memory or inefficiencies in scenarios where circular traversal would be more appropriate.
Summary
- Single Circular Linked List: Best used in scenarios where circular traversal or round-robin operations are needed. It simplifies certain operations but introduces complexity in handling circular links and potential infinite loops.
- Singly Linked List: More straightforward and easier to implement, suitable for applications requiring linear traversal and where circular behavior is not needed.
The choice between a single circular linked list and a singly linked list depends on the specific requirements of the application and the operations that need to be performed.
Advantages and Disadvantages of a Double Circular linked list over a Double linked list
A doubly circular linked list and a doubly linked list are both variations of linked lists, but they have distinct characteristics and use cases. Here’s a comparison of their advantages and disadvantages:
Doubly Circular Linked List
Advantages:
- Bidirectional Traversal:
- Like a doubly linked list, a doubly circular linked list allows traversal in both forward and backward directions.
- Circular Nature:
- The list forms a loop where the last node points back to the first node. This can be useful for applications that require circular iteration or cyclic operations.
- Efficient for Certain Operations:
- Operations that involve circular traversal or looping (e.g., round-robin scheduling) can be more straightforward and efficient because you don’t need to check for the end of the list.
- Simplified List Management:
- Adding or removing elements from the head or tail of the list can be simplified, as there’s no need to handle end-of-list conditions.
Disadvantages:
- Complexity in Implementation:
- Managing circular references can complicate code, especially when performing insertion and deletion operations. Special care is needed to maintain the circular structure correctly.
- Infinite Loops Risk:
- Care must be taken to avoid infinite loops in traversal or other operations, as the list forms a continuous loop.
- Increased Overhead:
- The circular nature might introduce additional overhead in some cases, as operations that assume a non-circular structure need extra handling.
Doubly Linked List
Advantages:
- Flexible Traversal:
- Supports traversal in both forward and backward directions, allowing for efficient access to both ends of the list.
- Simpler Management:
- Easier to understand and implement compared to circular lists because it doesn’t involve circular references. You can easily identify the beginning and end of the list.
- Versatile Use Cases:
- Suitable for a wide range of applications where circular traversal is not required. Useful for many standard data structures and algorithms.
Disadvantages:
- End-of-List Handling:
- Special cases need to be handled for operations at the end of the list (e.g., detecting the end of traversal or insertion/deletion at the end).
- Less Efficient for Circular Operations:
- For use cases requiring circular iteration or cyclic behavior, a doubly circular linked list might be more efficient.
- Extra Space Overhead:
- Each node requires extra space for two pointers (previous and next), which can be a consideration in memory-constrained environments.
Summary
- Doubly Circular Linked List: Best for scenarios where you need continuous circular traversal and where circular behavior simplifies your operations. It introduces some complexity in implementation and can lead to infinite loops if not managed carefully.
- Doubly Linked List: More straightforward to implement and understand, and suitable for general-purpose use where circular behavior is not required. It may require more handling for operations at the ends of the list and can be less efficient for circular operations.