What is C language
C language is a high-level programming language developed by Dennis Ritchie at Bell Labs in the early 1970s. It is widely used for system programming, developing operating systems, and writing applications that require performance. and the people choose the c programming the main reason it is easy to understand when we compare to other programming language.
we will use C language in
Operating Systems: The Unix operating system and its derivatives (like Linux) are written in C.
Embedded Systems: Firmware for devices like routers, appliances, and automotive systems is often developed in C
Game Development: Some game engines and performance-critical components are written in C for speed and efficiency
Compilers and Interpreters: Many compilers and interpreters for other programming languages are written in C.
C is the widely used language.it provides many features that are given below:
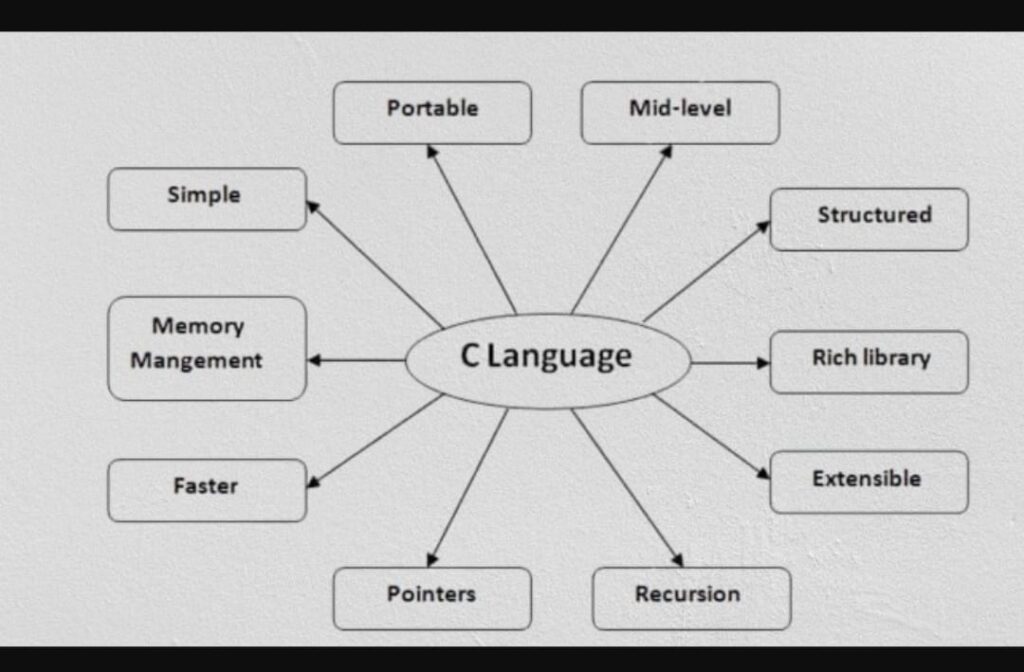
History or Evolution of c language
- ALGOL (1960): Known as the “father” of programming languages, ALGOL introduced the concept of structured programming. It was widely used in Europe and set the stage for future languages.
- BCPL (1967): Developed by Martin Richards, BCPL (Basic Combined Programming Language) was designed as a general-purpose language. It influenced later languages, including C.
- B (1970): Created by Ken Thompson, B was inspired by BCPL. It was used to develop the early UNIX operating system at AT&T and Bell Labs.
- C (1972): Dennis Ritchie and Ken Thompson at Bell Labs developed C, combining elements from ALGOL, BCPL, and B. C quickly became popular due to its efficiency and power and played a key role in the development of UNIX.
- ANSI C (1989): To standardize C and ensure it worked consistently across different systems, the American National Standards Institute (ANSI) introduced a standard for C. This was later approved by the International Standards Organization (ISO) in 1990, making the language known as “ANSI C”. This standardization helped C gain widespread use and compatibility across various platforms.
In summary, C built upon earlier languages and innovations, becoming a powerful and influential language in its own right.
Structure of C program
- Preprocessor Directives:
- These lines start with # and are processed before the compilation begins.
Example: #include
Purpose: Includes header files necessary for standard functions.
2.Function Definitions:
- Every C program must have a main function, which is the entry point of the program.
Example:int main() {
// code
return 0;
}
Purpose: This is where the execution of the program starts.
- Variable Declarations:
- Variables must be declared before they are used.
Example:
int age;
Purpose: Specifies the type and name of variables to be used.
- Statements and Expressions:
The main logic of the program is written here, including calculations, input/output operations, and control flow.
Example:printf("Hello, World!n");
int sum = a + b;
//Purpose: Executes the actual functionality of the program.
Comments:
- Comments are included to explain the code and are ignored by the compiler.
Example:
// This is a single-line comment
/*
This is a multi-line comment
*/ - Purpose: Improves code readability and maintainability.
Example of C program
#include<stdio.h> // Preprocessor directive to include standard I/O functions
// Main function where the execution of the program begins
int main() {
// Variable declaration
int number;
// Output statement
printf(“Enter an integer: “);
// Input statement
scanf(“%d”, &number);
// Output the entered number
printf(“You entered: %dn”, number);
// Return statement
return 0; // Indicates that the program ended successfully
}
Breakdown of the Example
- Preprocessor Directive: #include includes the standard input/output library needed for printf and scanf.
- Main Function: int main() is where the program starts executing. It’s required in every C program.
- Variable Declaration: int number; declares an integer variable named number.
- Statements:
- printf(“Enter an integer: “); prints a message to the console.
- scanf(“%d”, &number); reads an integer from the user and stores it in number.
- printf(“You entered: %dn”, number); prints the value entered by the user.
- Return Statement: return 0; signifies that the program has executed successfully and returns control to the operating system.
Explain the steps involved in Editing,Compling,Execting and Debugging of c program
Editing:
In C programming, editing typically refers to making changes to the code. This could involve:
- Modifying Code: Adding, deleting, or changing lines of code to improve functionality or fix bugs.
- Formatting: Adjusting the layout of the code to improve readability, such as proper indentation and spacing.
- Refactoring: Restructuring existing code without changing its external behavior to improve its structure and efficiency.
For example, if you have a simple C program:
#include<stdio.h>
int main()
{
printf(“Hello, bhanu”);
return 0;
}
Editing this code might involve changing the message:
#include<stdio.h>
int main()
{
printf("Hello, prakashn"); // Changed from "Hello, bhanu" to "Hello, prakash"
return 0;
}
int main()
printf("Hello, prakashn"); // Changed from "Hello, bhanu" to "Hello, prakash"
return 0;
}
what is compilation
The compilation is a process of converting the source code into object code. It is done with the help of the compiler. The compiler checks the source code for the syntactical or structural errors, and if the source code is error-free, then it generates the object code.

The c compilation process converts the source code taken as input into the object code or machine code. The compilation process can be divided into four steps, i.e., Pre-processing, Compiling, Assembling, and Linking.
Executing
- Involves running the compiled C program to see its output and functionality.
- After successful compilation (generating an executable file), use the terminal to execute the program.
- The command typically follows the format ./program_name, where program_name is the name you assigned during compilation (e.g., ./hello bhanu).
- If your program runs correctly, you’ll observe the expected output on the console.
Debugging:
- Comes into play when your program doesn’t produce the expected output or crashes.
- Aims to identify and fix errors (bugs) in your C code.
- Common techniques include:
- Printing statements: Insert printf statements to print variable values at different code sections to track execution flow and pinpoint issues.
- Debuggers: Tools like GDB allow you to set breakpoints, examine variables, and step through your code line by line to isolate errors.
- Mental debugging: Carefully review your code for logical errors (typos, incorrect operators, missing conditions).
- Involves fixing the identified errors, recompiling, and retesting the program until it functions as intended.
Describe character set,c-tokens ,keywords ,Identifiers ,constants,variables
character set:
In C programming, the character set refers to the collection of all valid characters that can be used to create source code. These characters include:
- Uppercase and lowercase letters (A-Z, a-z)
- Digits (0-9)
- Special characters (!, @, #, $, %, ^, &, *, (, ), _, -, +, =, {, }, [, ], |, , :, ;, ‘, “, <, >, ,, ., ?, /etc.)
- Whitespace characters (space, tab, newline, etc.)
C primarily uses the ASCII (American Standard Code for Information Interchange) character set, which assigns a unique numerical value (ASCII value) to each character. This allows computers to represent and manipulate text data efficiently.
keywords
Keywords are predefined or reserved words that have special meanings to the compiler. These are part of the syntax and cannot be used as identifiers in the program. A list of keywords in C or reserved words in the C programming language supports 32 keywords are mentioned below:
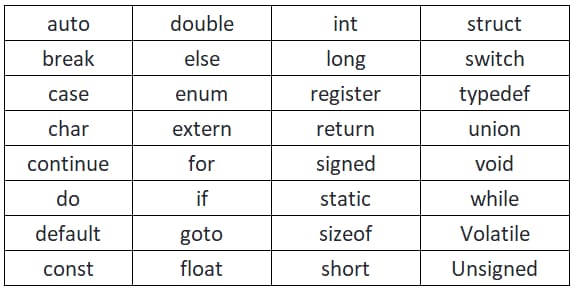
Here’s a list of C keywords and a brief description of each:
- auto: Defines automatic storage duration; typically used for local variables.
- break: Exits from the current loop or switch statement.
- case: Defines a branch in a switch statement.
- char: Defines a character data type.
- const: Defines a constant value that cannot be modified.
- continue: Skips the rest of the loop iteration and proceeds with the next iteration.
- default: Specifies the default branch in a switch statement if no case matches.
- do: Starts a do-while loop, which executes at least once before checking the condition.
- double: Defines a double-precision floating-point data type.
- else: Specifies an alternative branch in an if-else statement.
- enum: Defines a set of named integer constants.
- extern: Declares a variable or function defined in another file or translation unit.
- float: Defines a single-precision floating-point data type.
- for: Starts a for loop.
- goto: Transfers control to a specified label (generally discouraged).
- if: Starts a conditional statement.
- int: Defines an integer data type.
- long: Defines a long integer data type.
- register: Suggests that the variable be stored in a CPU register for faster access (compiler may ignore this).
- return: Exits from a function and optionally returns a value.
- short: Defines a short integer data type.
- signed: Specifies a signed integer data type (can represent both positive and negative values).
- sizeof: Returns the size of a data type or variable in bytes.
- static: Defines a variable or function with static storage duration; retains its value between function calls.
- struct: Defines a structure that groups related variables of different types.
- switch: Starts a switch statement for multi-way branching.
- typedef: Creates an alias for a data type.
- union: Defines a union that can store different data types in the same memory location.
- unsigned: Specifies an unsigned integer data type (can only represent positive values).
- void: Represents the absence of a value or data type; used for functions that do not return a value.
- volatile: Indicates that a variable’s value may change at any time without any action being taken by the code the compiler finds nearby.
- while: Starts a while loop, which continues as long as its condition is true.
Identifiers
Identifiers in C are names given to various program elements such as variables, functions, arrays, and structures. They help to identify and reference these elements in the code.
char section = ‘A’;
Rules to Name an Identifier in C
A programmer has to follow certain rules while naming variables. For the valid identifier, we must follow the given below set of rules.
Rules for Identifiers in C
- Allowed Characters:
- Identifiers can include letters (both lowercase a-z and uppercase A-Z) and digits (0-9).
- Special Characters:
- Identifiers cannot include special characters except for the underscore (_).
- No Spaces:
- Spaces are not allowed in identifiers.
- Starting Characters:
- An identifier must start with either a letter or an underscore. It cannot start with a digit.
- Keywords:
- You cannot use keywords as identifiers. Keywords are reserved words in C that have special meaning, such as int, char, struct, printf, and scanf. Using a keyword as an identifier will cause a compilation error.
- Uniqueness:
- Identifiers must be unique within their namespace. For example, you cannot have two variables with the same name within the same function.
- Case Sensitivity:
- C is case-sensitive, which means that name and NAME are considered different identifiers.
Examples
Valid Identifiers
int age; // ‘age’ is a valid identifier
float salary; // ‘salary’ is a valid identifier
char name[50]; // ‘name’ is a valid identifier
int _index; // ‘_index’ is a valid identifier
int studentID123; // ‘studentID123’ is a valid identifier
Invalid Identifiers
int 2ndNumber; // Invalid: Cannot start with a digit
float double; // Invalid: ‘double’ is a keyword
char new name; // Invalid: Cannot contain spaces
int %rate; // Invalid: Cannot contain special characters
Explanation
- int age;: Valid because it starts with a letter and includes only letters.
- float double;: Invalid because double is a keyword.
- char new name;: Invalid because it contains a space.
- int %rate;: Invalid because it includes a special character %.
Example Code
Here’s a simple example to show the use of valid identifiers:
#include<stdio.h>
// Function to calculate the sum of two integers
int calculateSum(int num1, int num2) {
return num1 + num2;
}
int main() {
int a = 5; // 'a' is a valid identifier
int b = 10; // 'b' is a valid identifier
int result; // 'result' is a valid identifier
result = calculateSum(a, b); // Using 'calculateSum' function
printf("Sum: %d\n", result); // Using 'result' identifier
return 0;
}
In this code:
- calculateSum, a, b, and result are identifiers .
Constants
The constants in C are the read-only variables whose values cannot be modified once they are declared in the C program. The type of constant can be an integer constant, a floating pointer constant, a string constant, or a character constant. In C language, the const keyword is used to define the constants.
Syntax to Define Constant
const data_type var_name = value;
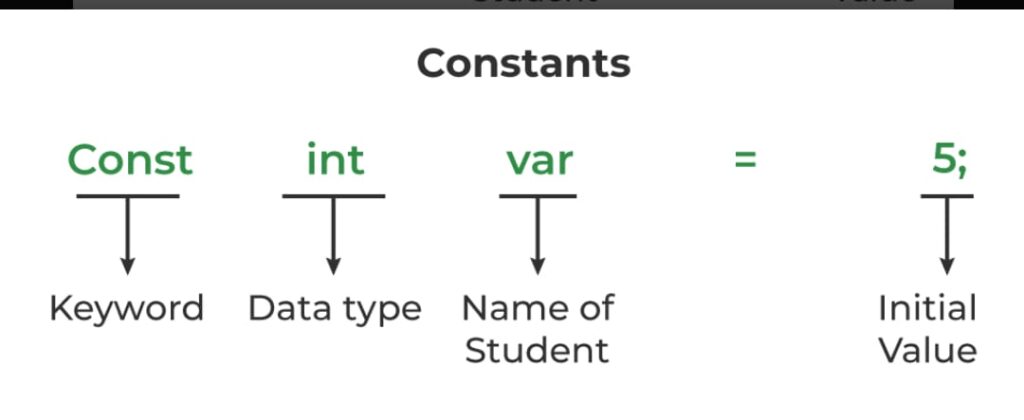
Variable
A variable in C language is the name associated with some memory location to store data of different types. There are many types of variables in C depending on the scope, storage class, lifetime, type of data they store, etc. A variable is the basic building block of a C program that can be used in expressions as a substitute in place of the value it stores.
Data types in c
In C programming, every variable has a specific type of data it can hold. This is called a “data type.” Data types tell the computer how much memory to set aside for a variable and what kind of operations can be done with it.
Data types are classsified into the following categories
1.primary Data types
2.user-defined data types
3.derived data types
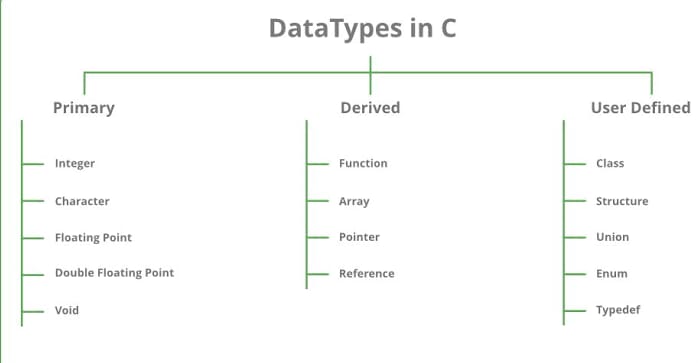
The following table provides the details of standard integer types with their storage sizes and value ranges.

Declaring (Creating) Variables
To create a variable, specify the type and assign it a value:
Syntax
type variableName = value;
Where type is one of C types (such as int), and variableName is the name of the variable (such as x or myName). The equal sign is used to assign a value to the variable.
Example
Create a variable called myNum of type int and assign the value 15 to it:
int myNum = 15;
You can also declare a variable without assigning the value, and assign the value later.
// Declare a variable
int myNum;
// Assign a value to the variable
myNum = 15;
User defined data types with a simple program
The data types that are defined by the user called the derived data types or user defined data types User-defined data types in C are primarily created using struct, union, and enum.
Here’s an example using a struct to define a simple data type representing a
student:Step-by-Step Example
1.Define the struct
2.Declare variables of the struct type
3.Initialize and use these variables
1.Structure (struct):
A struct (short for “structure”) is a user-defined data type that groups related variables of different types under a single name. Each variable in a structure is called a member. Structures are used to represent a record.
syantax:
struct bhanu
{
char name[50];
char city[2];
int pin;
2.Union :
A union is a user-defined data type that allows storing different data types in the same memory location. Unlike a structure, a union can only store one of its members at a time. The size of a union is determined by its largest member.
union bhanu
{
char name[50];
char city[2];
int pin;
2.class:
The building block of C++ that leads to Object-Oriented programming is a Class. It is a user-defined data type, which holds its own data members and member functions, which can be accessed and used by creating an instance of that class. A class is like a blueprint for an object.
syntax:
class bhanu
{
acces specifiers
data members;
member function()
{}
};