Define array
An array is a collection of similar data types stored in contiguous memory locations. In programming, an array of characters is referred to as a string. Each item in an array is called an element, and each element is stored at a unique memory location. Although all elements share the same variable name, they can be accessed individually using their index, also known as a subscript.
Arrays can be one-dimensional or multi-dimensional, depending on the number of subscripts used to access their elements. The indexing for arrays typically starts at zero. A one-dimensional array is called a vector, while a two-dimensional array is known as a matrix.
ADVANTAGES: array variable can store more than one value at a time where
other variable can store one value at a time
Example
Int a[20];
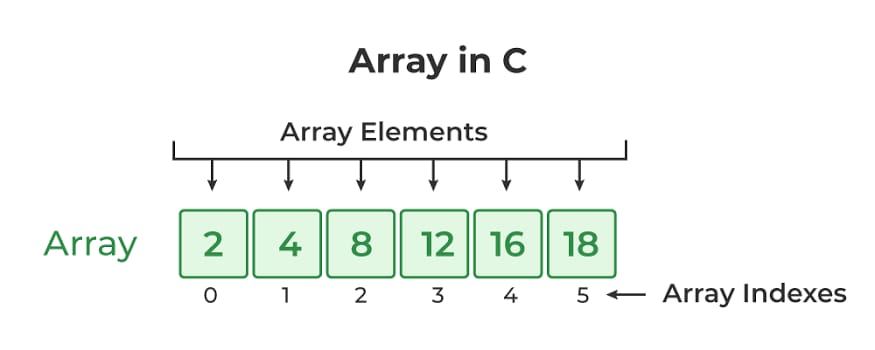
Types of array:
1.One dimensional arrays
2.Two dimensional arrays
3.Multidimensional arrays
1.One dimensional arrays:
The One-dimensional arrays, also known as 1-D arrays in C are those arrays that have only one dimension.
DECLARATION OF AN ONE DIMENSIONAL ARRAY :
Its syntax is :
Data type array name [size];
int arr[100];
int mark[100];
int a[5]={10,20,30,100,5}
The declaration of an array tells the compiler that, the data type, name of the array,
size of the array and for each element it occupies memory space.
Like for int data
type, it occupies 2 bytes for each element and for float it occupies 4 byte for each
element etc.
The size of the array operates the number of elements that can be
stored in an array and it may be a int constant or constant int expression.
We can represent individual array as :
int ar[5];
ar[0], ar[1], ar[2], ar[3], ar[4];
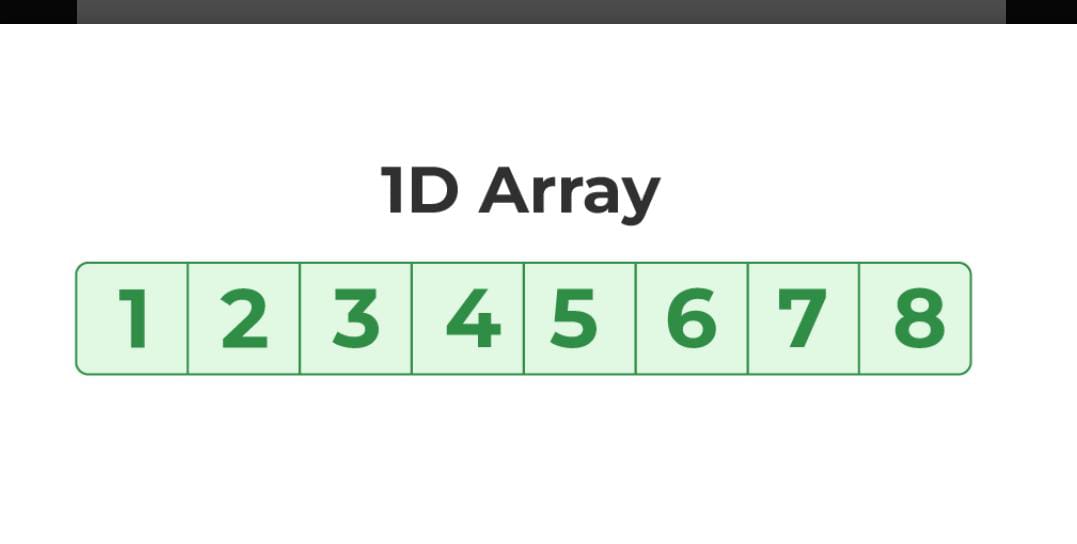
INITIALIZATION OF AN ARRAY:
After declaration element of local array has garbage value. If it is global or static
array then it will be automatically initialize with zero. An explicitly it can be
initialize that
Data type array name [size] = {value1, value2, value3…}
Example:
in ar[5]={20,60,90, 100,120}
C program to find highest value of an array
#include <stdio.h>
int main() {
int n, i;
int highest;
printf("Enter the number of elements in the array: ");
scanf("%d", &n);
int array[n];
printf("Enter %d elements:\n", n);
for (i = 0; i < n; i++) {
scanf("%d", &array[i]);
}
highest = array[0];
for (i = 1; i < n; i++) {
if (array[i] > highest) {
highest = array[i];
}
}
printf("The highest value in the array is: %d\n", highest);
return 0;
}
Explanation:
Input the number of elements: The user specifies how many elements the array will have.
Input elements of the array: The user enters each element of the array.
Find the highest value:
The program initializes highest with the first element and iterates through the array to find the maximum value.
Output the highest value:
The program prints the highest value found in the array.
C program to print Fibonacci series
#include <stdio.h>
int main() {
int n, i;
int a = 0, b = 1, next;
printf("Enter the number of terms in the Fibonacci series: ");
scanf("%d", &n);
printf("Fibonacci series up to %d terms:\n", n);
for (i = 0; i < n; i++) {
if (i == 0) {
printf("%d ", a);
} else if (i == 1) {
printf("%d ", b);
} else {
next = a + b;
a = b;
b = next;
printf("%d ", next);
}
}
printf("\n");
return 0;
}
Explanation:
Initialize Variables:
a and b are the first two terms of the Fibonacci series. next is used to compute subsequent terms.
Input Number of Terms:
The user specifies how many terms of the Fibonacci series to print.
Print Series:
Using a loop, the program prints each term of the Fibonacci series up to the specified number of terms.
Update Terms:
In each iteration of the loop, the next term is computed as the sum of a and b, and then a and b are updated for the next iteration.
Accessing the Elements in the Array:
Access Array Elements
We can access any element of an array in C using the array subscript operator [ ] and the index value i of the element.
array_name [index];
One thing to note is that the indexing in the array always starts with 0, i.e., the first element is at index 0 and the last element is at N – 1 where N is the number of elements in the array.
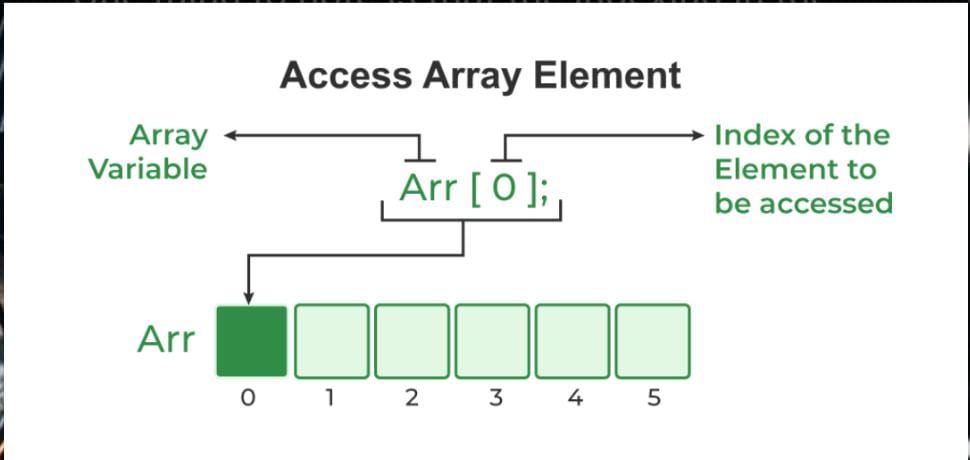
2.Two-Dimensional:
A Two-Dimensional (2D) array in C can be thought of as a grid or table, where you have rows and columns. Imagine a spreadsheet where each cell can hold a value. In C, a 2D array organizes data in this way, allowing you to access each piece of data by specifying its row and column.For example, if you have a 2D array called matrix with 3 rows and 4 columns, it can be visualized like this:
For example, if you have a 2D array called matrix with 3 rows and 4 columns, it can be visualized like this:
matrix[0][0] matrix[0][1] matrix[0][2] matrix[0][3]
matrix[1][0] matrix[1][1] matrix[1][2] matrix[1][3]
matrix[2][0] matrix[2][1] matrix[2][2] matrix[2][3]
Syntax of 2D Array in C
array_name[size1] [size2];
Here,
size1: Size of the first dimension.
size2: Size of the second dimension.
Initialization of 2-d array:
2-D array can be initialized in a way similar to that of 1-D array.
for example:-
int mat[4][3]={11,12,13,14,15,16,17,18,19,20,21,22};
These values are assigned to the elements row wise, so the values of
elements after this initialization are
Mat[0][0]=11, Mat[1][0]=14, Mat[2][0]=17 Mat[3][0]=20
Mat[0][1]=12, Mat[1][1]=15, Mat[2][1]=18 Mat[3][1]=21
Mat[0][2]=13, Mat[1][2]=16, Mat[2][2]=19 Mat[3][2]=22
Addition of two matrices in c:
#include <stdio.h>
#define ROWS 3
#define COLS 4
int main() {
int matrix1[ROWS][COLS] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
int matrix2[ROWS][COLS] = {
{12, 11, 10, 9},
{8, 7, 6, 5},
{4, 3, 2, 1}
};
int result[ROWS][COLS];
// Add the matrices
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
result[i][j] = matrix1[i][j] + matrix2[i][j];
}
}
// Print the result
printf("Resultant Matrix:\n");
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
printf("%d ", result[i][j]);
}
printf("\n");
}
return 0;
}
Explanation:
Matrix Declaration:
matrix1 and matrix2 are the two matrices to be added.
result is the matrix to store the result of the addition.
Matrix Initialization:
The matrices are initialized with predefined values.
Matrix Addition:The nested for loops iterate over each element of the matrices, adding corresponding elements and storing the result in the result matrix.
Printing the Result:Another set of nested for loops prints the resultant matrix row by row.
Notes:
Ensure that both matrices have the same dimensions; otherwise, matrix addition is not possible.Adjust ROWS and COLS as needed for different matrix sizes.
Multiplication of two matrices in c:
#include <stdio.h>
#define ROWS1 2
#define COLS1 3
#define ROWS2 3
#define COLS2 2
int main() {
int matrix1[ROWS1][COLS1] = {
{1, 2, 3},
{4, 5, 6}
};
int matrix2[ROWS2][COLS2] = {
{7, 8},
{9, 10},
{11, 12}
};
int result[ROWS1][COLS2] = {0}; // Initialize result matrix to 0
// Matrix multiplication
for (int i = 0; i < ROWS1; i++) {
for (int j = 0; j < COLS2; j++) {
result[i][j] = 0; // Initialize result element to 0
for (int k = 0; k < COLS1; k++) {
result[i][j] += matrix1[i][k] * matrix2[k][j];
}
}
}
// Print the result
printf("Resultant Matrix:\n");
for (int i = 0; i < ROWS1; i++) {
for (int j = 0; j < COLS2; j++) {
printf("%d ", result[i][j]);
}
printf("\n");
}
return 0;
}
Explanation:
Matrix Declaration:
matrix1 and matrix2 are the matrices to be multiplied.
result is the matrix to store the result of the multiplication.
Matrix Initialization:
The matrices are initialized with predefined values.
Matrix Multiplication:The outer for loops iterate over each element of the result matrix.The inner for loop performs the dot product of the corresponding row from matrix1 and column from matrix2.
Printing the Result:
A nested for loop prints the resultant matrix row by row.
Notes:
For matrix multiplication to be valid, the number of columns in the first matrix (COLS1) must be equal to the number of rows in the second matrix (ROWS2).Adjust ROWS1, COLS1, ROWS2, and COLS2 as needed for different matrix sizes.
Define string in c
String
Array of character is called a string. It is always terminated by the NULL
character.
String is a one dimensional array of character.and string also known as collection of a characters
We can initialize the string as
char name[]={‘b’,’h’,’a’,’n’,’u’\o’};
Here each character occupies 1 byte of memory and last character is always NULL
character. Where ’\o’ and 0 (zero) are not same, where ASCII value of ‘\o’ is 0
and ASCII value of 0 is 48. Array elements of character array are also stored in
contiguous memory allocation.
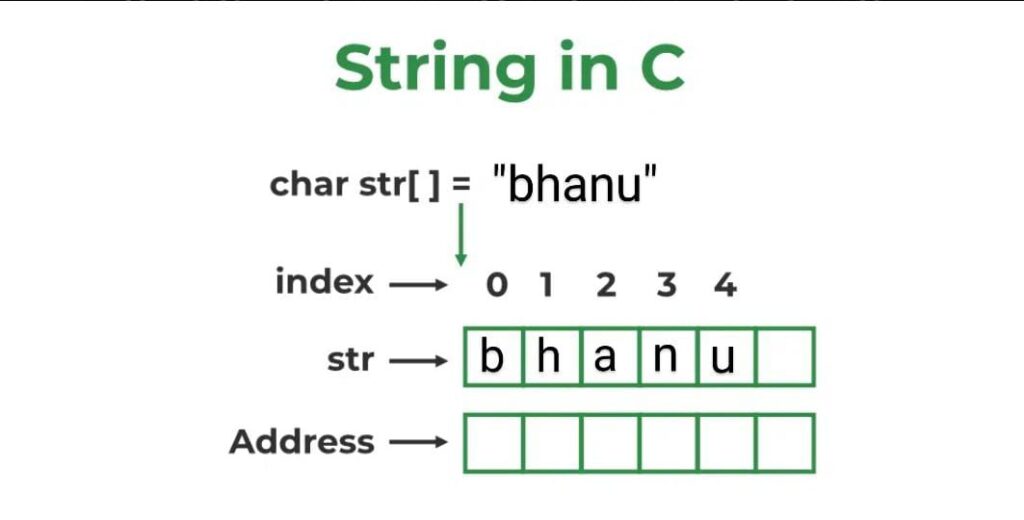
C String Declaration Syntax
Declaring a string in C is as simple as declaring a one-dimensional array. Below is the basic syntax for declaring a string.
char string_name[size];
In the above syntax string_name is any name given to the string variable and size is used to define the length of the string, i.e the number of characters strings will store.
C String Initialization
A string in C can be initialized in different ways. We will explain this with the help of an example. Below are the examples to declare a string with the name str and initialize it with “bhanuprakash”.
Assigning a String Literal:
String literals are often allocated with a specific size, and it is important to account for an additional space for the null character (‘\0’), which terminates the string. Therefore, to store a string of length n, the array should be declared with a size of at least n + 1. This extra space ensures that there is room for the null terminator.
char str[50] = “bhanu”;
Note:
When a Sequence of characters enclosed in the double quotation marks is encountered by the compiler, a null character ‘\0’ is appended at the end of the string by default.
C string Example:
#include <stdio.h>
#include <string.h>
int main()
{
char str[] = "bhanu";
printf("%s\n", str);
int length = 0;
length = strlen(str);
printf("Length of string str is %d", length);
return 0;
}
Read a String Input From the User
The following example demonstrates how to take string input using scanf() function in C:
#include<stdio.h>
int main()
{
// declaring string
char str[50];
// reading string
scanf("%s",str);
// print string
printf("%s",str);
return 0;
}
How to Read a String Separated by Whitespaces in C?
We can use multiple methods to read a string separated by spaces in C. The two of the common ones are:
We can use the fgets() function to read a line of string and gets() to read characters from the standard input (stdin) and store them as a C string until a newline character or the End-of-file (EOF) is reached.
We can also scanset characters inside the scanf() function
Example of String Input using gets()L
#include <stdio.h>
int main() {
char str[100]; // Declare a character array (string) of size 100
printf("Enter a string: ");
gets(str); // Read a string from standard input
printf("You entered: %s\n", str); // Print the entered string
return 0;
}
String library function:
There are several string library functions used to manipulate string and the
prototypes for these functions are in header file “string.h”. Several string functions
are.
strlen():
This function return the length of the string. i.e. the number of characters in the
string excluding the terminating NULL character.
It accepts a single argument which is pointer to the first character of the string.
strcmp():
This function is used to compare two strings. If the two string match, strcmp()
return a value 0 otherwise it return a non-zero value. It compare the strings
character by character and the comparison stops when the end of the string is
reached or the corresponding characters in the two string are not same.
Strcpy():
This function is used to copying one string to another string. The function
strcpy(str1,str2) copies str2 to str1 including the NULL character. Here str2 is the
source string and str1 is the destination string.
The old content of the destination string str1 are lost. The function returns a pointer
to destination string str1.
Strcat():
This function is used to append a copy of a string at the end of the other string. If
the first string is “”Purva” and second string is “Belmont” then after using this
function the string becomes “PusvaBelmont”. The NULL character from str1 is
moved and str2 is added at the end of str1. The 2nd string str2 remains unaffected.
A pointer to the first string str1 is returned by the function.
Here is the example program
For Strlen(), Strcmp(),Strcpy(),Strcat():
#include <stdio.h>
#include <string.h>
int main() {
char str1[50] = "Hello"; // Initial string
char str2[] = "World!";
char str3[50]; // Buffer to hold copied string
size_t length;
// Copy str1 to str3
strcpy(str3, str1);
printf("Copied String: %s\n", str3); // Output: "Hello"
// Concatenate str2 to str3
strcat(str3, str2);
printf("Concatenated String: %s\n", str3); // Output: "HelloWorld!"
// Find the length of the concatenated string
length = strlen(str3);
printf("Length of Concatenated String: %zu\n", length); // Output: 12
// Compare str1 and str2
int cmpResult = strcmp(str1, str2);
if (cmpResult < 0) {
printf("\"%s\" is less than \"%s\"\n", str1, str2);
} else if (cmpResult > 0) {
printf("\"%s\" is greater than \"%s\"\n", str1, str2);
} else {
printf("\"%s\" is equal to \"%s\"\n", str1, str2);
}
return 0;
}
Explanation:
strcpy(str3, str1);:Copies the content of str1 into str3.
strcat(str3, str2);:
Appends str2 to the end of str3.strlen(str3);:
Calculates the length of the concatenated string str3.
strcmp(str1, str2);:
Compares str1 with str2 and prints the result.
Structure in c
In C, a structure (or struct) is a user-defined data type that groups together variables under a single name. These variables can be of different types and are referred to as members of the structure. Structures are used to represent a record or a complex data entity in a program.
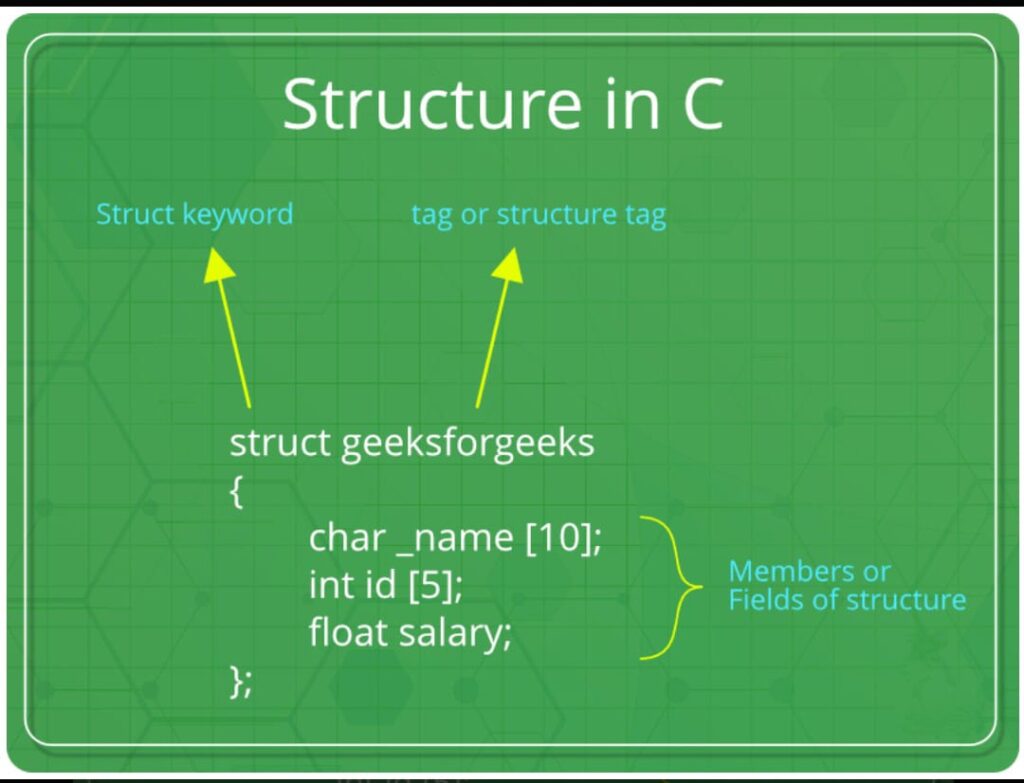
Defining a Structure
To define a structure, use the struct keyword followed by a block of code that specifies the members of the structure. Here’s a basic example:
Uses of Structure in C
C structures are used for the following:
The structure can be used to define the custom data types that can be used to create some complex data types such as dates, time, complex numbers, etc. which are not present in the language.
It can also be used in data organization where a large amount of data can be stored in different fields.
Structures are used to create data structures such as trees, linked lists, etc.
They can also be used for returning multiple values from a function.
Syntax
struct structure_name {
data_type member_name1;
data_type member_name1;
….
….
};
The above syntax is also called a structure template or structure prototype and no memory is allocated to the structure in the declaration.
Example program on structure:
#include <stdio.h>
// Define a structure named 'Person'
struct Person {
char name[50];
int age;
float height;
};
int main() {
// Declare a variable of type 'Person'
struct Person person1;
// Initialize the members of the structure
person1.age = 30;
person1.height = 5.9;
snprintf(person1.name, sizeof(person1.name), "bhanu");
// Access and print the members of the structure
printf("Name: %s\n", person1.name);
printf("Age: %d\n", person1.age);
printf("Height: %.1f\n", person1.height);
return 0;
}
Explanation:
Structure Definition:struct Person {
char name[50];
int age;
float height;
};
Defines a structure named Person with three members: name, age, and height.
Structure Variable Declaration:struct Person person1;
Declares a variable person1 of type struct Person.Accessing and Initializing Members:
person1.age = 30;
person1.height = 5.9;
snprintf(person1.name, sizeof(person1.name), "bhanu");Sets the values for each member of the structure.Accessing Members:printf("Name: %s\n", person1.name);
printf("Age: %d\n", person1.age);
printf("Height: %.1f\n", person1.height);
Accesses and prints the values of each member.
Structure Initialization
You can also initialize a structure when declaring it:
struct Person person2 = {"prakash", 28, 5.5};
In this case, person2 is initialized with "Prakash" for name, 28 for age, and 5.5 for height.
Nested Structure :
In C, nesting structures involves including one structure as a member of another structure. This process allows you to build more complex data types by combining simpler ones. Nested structures enable you to group related data together in a hierarchical manner.
Example:
// Define the inner structure
struct InnerStruct {
// Members of InnerStruct
type member1;
type member2;
};
// Define the outer structure that includes InnerStruct
struct OuterStruct {
// Members of OuterStruct
type member1;
type member2;
struct InnerStruct inner; // Nested structure
};
Example program on Nested structure:
#include <stdio.h>
// Forward declaration of the Address structure
struct Address;
// Define the Person structure that uses the Address structure
struct Person {
char name[50];
int age;
struct Address *address; // Pointer to the Address structure
};
// Define the Address structure after Person
struct Address {
char street[100];
char city[50];
int zip;
};
int main() {
// Initialize and use the structures
struct Address addr = {"123 Elm Street", "Springfield", 12345};
struct Person person = {"bhanu", 30, &addr}; // Using pointer to Address
printf("Name: %s\n", person.name);
printf("Age: %d\n", person.age);
printf("Address: %s, %s %d\n", person.address->street, person.address->city, person.address->zip);
return 0;
}
In C, you can create arrays of structures to handle multiple instances of a data structure efficiently. This is useful when you need to manage a collection of similar objects, such as a list of students or employees.
Syntax for Array of Structures
Define a Structure:
First, define the structure that will be used to create the array.
struct Person {
char name[50];
int age;
float height;
};
Declare an Array of Structures:
Declare an array where each element is of the type of the defined structure.
struct Person people[10]; // Array of 10 Person structures
Example:
#include <stdio.h>
#define SIZE 3
// Define the structure
struct Person {
char name[50];
int age;
float height;
};
int main() {
// Declare and initialize an array of structures
struct Person people[SIZE] = {
{"Alice", 28, 5.5},
{"Bob", 35, 6.0},
{"Charlie", 22, 5.8}
};
// Access and print the data from the array of structures
for (int i = 0; i < SIZE; i++) {
printf("Person %d:\n", i + 1);
printf("Name: %s\n", people[i].name);
printf("Age: %d\n", people[i].age);
printf("Height: %.1f\n", people[i].height);
printf("\n");
}
return 0;
}
Explanation:
Structure Definition:struct Person {
char name[50];
int age;
float height;
};
This defines a structure named Person with three members:
name, age, and height.
Array Declaration and Initialization:struct Person people[SIZE] = {
{"Alice", 28, 5.5},
{"Bob", 35, 6.0},
{"Charlie", 22, 5.8}
};
Here, people is an array of Person structures with 3 elements, each initialized with specific values.
Accessing and Printing Array Elements:
for (int i = 0; i < SIZE; i++) {
printf("Person %d:\n", i + 1);
printf("Name: %s\n", people[i].name);
printf("Age: %d\n", people[i].age);
printf("Height: %.1f\n", people[i].height);
printf("\n");
}
This loop iterates through the people array and prints the details of each Person.
Summary
Structure Definition: Define a structure with required members.
Array Declaration: Create an array where each element is of the structure type.
Initialization and Access: Initialize the array and access its elements using the array index.
Union in c
Union is derived data type contains collection of different data type or dissimilar
elements. All definition declaration of union variable and accessing member is
similar to structure, but instead of keyword struct the keyword union is used, the
main difference between union and structure is Each member of structure occupy the memory location, but in the unions
members share memory. Union is used for saving memory and concept is useful
when it is not necessary to use all members of union at a time.
Where union offers a memory treated as variable of one type on one occasion
where (struct), it read number of different variables stored at different place of
memory.
Uses of Unions
Memory Efficiency: Unions can save memory by using the same memory location for multiple data types.
This is useful in memory-constrained environments.
Variant Data Types:
Unions are used when a variable can hold one of several data types, such as in implementing a variant data type or a type that can hold multiple formats.
Type-Punning: Unions can be used to access the same memory in different ways, known as type-punning. This can be useful in low-level programming and hardware interfacing.
Protocols and Data Conversion: Unions are often used in network protocols and file formats where data is read as different types depending on the context.
Summary
Union Definition: Use union to define a union with multiple members.
Memory Sharing: All members of a union share the same memory location.
Last Stored Value: Only the last stored value is valid;
accessing other members may result in undefined data.
Uses: Memory efficiency, variant data types, type-punning, and data conversion.
Syntax of Union in C
The syntax of the union in C can be divided into three steps which are as follows:
C Union Declaration
In this part, we only declare the template of the union, i.e., we only declare the members’ names and data types along with the name of the union. No memory is allocated to the union in the declaration.
Note:
Keep in mind that we have to always end the union declaration with a semi-colon.
Syntax
union union_name {
datatype member1;
datatype member2;
…
};
Example program on union
#include <stdio.h>
// Define a union
union Data {
int i;
float f;
char str[20];
};
int main() {
union Data data;
// Store an integer
data.i = 10;
printf("data.i: %d\n", data.i);
// Store a float
data.f = 220.5;
printf("data.f: %.2f\n", data.f);
// Store a string
snprintf(data.str, sizeof(data.str), "Hello, World!");
printf("data.str: %s\n", data.str);
// Accessing the members after storing different types
// Only the last stored member will be valid
printf("data.i after storing str: %d\n", data.i);
printf("data.f after storing str: %.2f\n", data.f);
return 0;
}
Difference between structure and union:
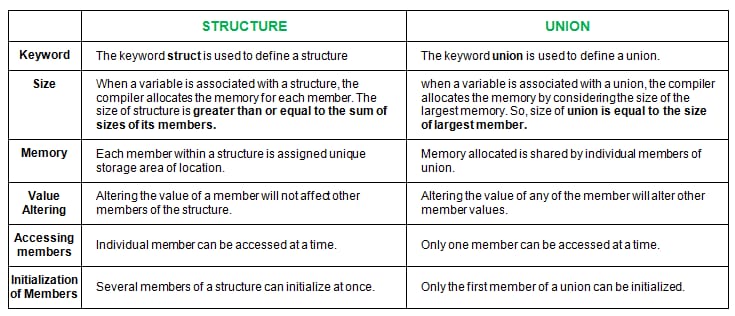